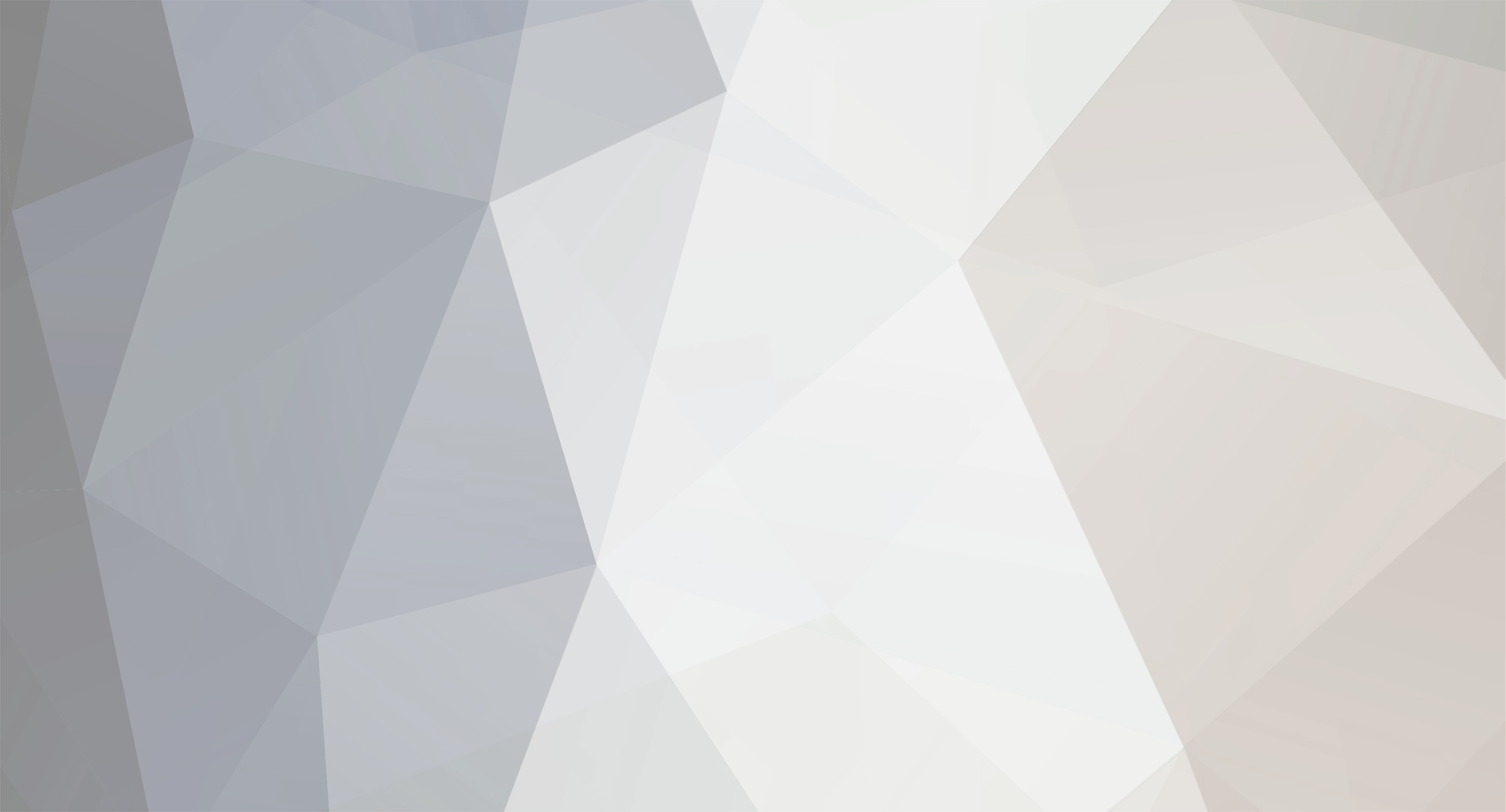
enneract
Active Members-
Posts
24 -
Joined
-
Last visited
Everything posted by enneract
-
The first while loop should only execute for a few fractions of a second, it just does the initial hiding.
-
It's auto-hiding functionality is based on mouse cursor proximity, which is utterly useless for what I am using it as. Using it as an application launcher for my tablet PC, and if I am using the touch screen, it is nearly impossible to trigger. If you ask why I don't just use the win7 taskbar - it is for a similar reason, that in portrait mode, it is extremely difficult to hit the UI controls to change the taskbar 'page' using touch.
-
It monitors for visibility of a dock program called RocketDock, and sends a hotkey to hide it after it has been visible to a certain amount of time, or if a left click occurs. As for the sleep within the callback function... I can't find any way around it. Without it, the dock often doesn't register the click before the script hides the window. I seemed to have worked around it by creating the hook when the window becomes visible, and destroying it when the window is hidden, but it does create a slight delay where mouse clicks can't be detected.
-
Can anyone spot where I have a problem that could introduce a major memory leak or other problem causing severe performance degradation? My system drops to a crawl about 60 seconds after launching the script. The other problem is that the mouse hook only seems to trigger on the first left click after the script is run, and I don't have a clue about this either. ; ~~ Mouse Hook ~~ ;For more info, Visit: http://msdn.microsoft.com/en-us/library/ms644986(VS.85).aspx ;Include GUI Consts #include <GUIConstants.au3> ;for $GUI_EVENT_CLOSE #Include <WinAPI.au3> ;for HIWORD #NoTrayIcon ;These constants found in the helpfile under Windows Message Codes Global Const $WM_LBUTTONDOWN = 0x0201 Global Const $timeout = 5 ;seconds ;Consts/structs from msdn Global Const $MSLLHOOKSTRUCT = $tagPOINT & ";dword mouseData;dword flags;dword time;ulong_ptr dwExtraInfo" ;~ Global Const $WH_MOUSE_LL = 14 ;already declared ;~ Global Const $tagPOINT = "int X;int Y" ;already declared ;Register callback $hKey_Proc = DllCallbackRegister("_Mouse_Proc", "int", "int;ptr;ptr") $hM_Module = DllCall("kernel32.dll", "hwnd", "GetModuleHandle", "ptr", 0) $hM_Hook = DllCall("user32.dll", "hwnd", "SetWindowsHookEx", "int", $WH_MOUSE_LL, "ptr", DllCallbackGetPtr($hKey_Proc), "hwnd", $hM_Module[0], "dword", 0) $timer = 0 Run("C:\Program Files\RocketDock\RocketDock.exe") ;launch RocketDock ;ConsoleWrite("Stage 1 ") While 1 If BitAND(WinGetState("RocketDock"),2) Then ;Wait for window to appear Send("!^r") ;close dock ;ConsoleWrite("Stage 3 ") ExitLoop EndIf ;ConsoleWrite("Stage 2 ") WEnd While 1 If BitAND(WinGetState("RocketDock"), 2) Then ;if visible $timer = TimerInit() ;start timer While BitAND(WinGetState("RocketDock"), 2) ;while visible If TimerDiff($timer) > $timeout * 1000 Then ;check TimerDiff send("!^r") ;ConsoleWrite("Stage 6 ") EndIf ;ConsoleWrite("Stage 5 ") Sleep(500) WEnd EndIf ;ConsoleWrite("Stage 4 ") Sleep(1000) ;one second pause, this isn't high priority WEnd Exit Func _Mouse_Proc($nCode, $wParam, $lParam) ;function called for mouse events.. If $nCode < 0 OR $wParam <> $WM_LBUTTONDOWN Then ;recommended, see http://msdn.microsoft.com/en-us/library/ms644986(VS.85).aspx $ret = DllCall("user32.dll", "long", "CallNextHookEx", "hwnd", $hM_Hook[0], _ "int", $nCode, "ptr", $wParam, "ptr", $lParam) ;recommended Return $ret[0] EndIf ConsoleWrite("Stage 7 ") If BitAND(WinGetState("RocketDock"), 2) Then Sleep(500) ConsoleWrite("Stage 8 ") Send("!^r") EndIf ;This is recommended instead of Return 0 $ret = DllCall("user32.dll", "long", "CallNextHookEx", "hwnd", $hM_Hook[0], _ "int", $nCode, "ptr", $wParam, "ptr", $lParam) Return $ret[0] EndFunc ;==>_Mouse_Proc Func OnAutoItExit() DllCall("user32.dll", "int", "UnhookWindowsHookEx", "hwnd", $hM_Hook[0]) $hM_Hook[0] = 0 DllCallbackFree($hKey_Proc) $hKey_Proc = 0 EndFunc ;==>OnAutoItExit
-
Not really sure what I have here, or where to go with it. I'd like to use an autoit script to manage certain proprietary buttons on my laptop, and I have the source to a linux driver which documents the 'event GUID' as well as what appear to be offsets for the individual key events. Can someone point me in the direction of what I need to learn in order to utilize this? /* * Dell WMI hotkeys * * Copyright (C) 2008 Red Hat <mjg@redhat.com> * * Portions based on wistron_btns.c: * Copyright (C) 2005 Miloslav Trmac <mitr@volny.cz> * Copyright (C) 2005 Bernhard Rosenkraenzer <bero@arklinux.org> * Copyright (C) 2005 Dmitry Torokhov <dtor@mail.ru> * * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program; if not, write to the Free Software * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA */ #include <linux/kernel.h> #include <linux/module.h> #include <linux/init.h> #include <linux/types.h> #include <linux/input.h> #include <acpi/acpi_drivers.h> #include <linux/acpi.h> #include <linux/string.h> MODULE_AUTHOR("Matthew Garrett <mjg@redhat.com>"); MODULE_DESCRIPTION("Dell laptop WMI hotkeys driver"); MODULE_LICENSE("GPL"); #define DELL_EVENT_GUID "9DBB5994-A997-11DA-B012-B622A1EF5492" MODULE_ALIAS("wmi:"DELL_EVENT_GUID); struct key_entry { char type; /* See KE_* below */ u16 code; u16 keycode; u8 state; }; enum { KE_KEY, KE_SW, KE_IGNORE, KE_END }; /* * Certain keys are flagged as KE_IGNORE. All of these are either * notifications (rather than requests for change) or are also sent * via the keyboard controller so should not be sent again. */ static struct key_entry dell_wmi_keymap[] = { {KE_KEY, 0xe045, KEY_PROG1}, {KE_SW , 0xe046, SW_TABLET_MODE,1}, /* Going to tablet mode */ {KE_SW , 0xe047, SW_TABLET_MODE,0}, /* Coming from tablet mode */ {KE_KEY, 0xe009, KEY_EJECTCD}, /* These also contain the brightness level at offset 6 */ {KE_KEY, 0xe006, KEY_BRIGHTNESSUP}, {KE_KEY, 0xe005, KEY_BRIGHTNESSDOWN}, /* Battery health status button */ {KE_KEY, 0xe007, KEY_BATTERY}, /* This is actually for all radios. Although physically a * switch, the notification does not provide an indication of * state and so it should be reported as a key */ {KE_KEY, 0xe008, KEY_WLAN}, /* The next device is at offset 6, the active devices are at offset 8 and the attached devices at offset 10 */ {KE_KEY, 0xe00b, KEY_DISPLAYTOGGLE}, {KE_KEY, 0xe00c, KEY_KBDILLUMTOGGLE}, /* BIOS error detected */ {KE_KEY, 0xe00d, KEY_RESERVED}, /* Wifi Catcher */ {KE_KEY, 0xe011, KEY_PROG2}, /* Ambient light sensor toggle */ {KE_KEY, 0xe013, KEY_RESERVED}, {KE_KEY, 0xe020, KEY_MUTE}, {KE_KEY, 0xe02e, KEY_VOLUMEDOWN}, {KE_KEY, 0xe030, KEY_VOLUMEUP}, {KE_KEY, 0xe033, KEY_KBDILLUMUP}, {KE_KEY, 0xe034, KEY_KBDILLUMDOWN}, {KE_KEY, 0xe03a, KEY_CAPSLOCK}, {KE_KEY, 0xe045, KEY_NUMLOCK}, {KE_KEY, 0xe046, KEY_SCROLLLOCK}, {KE_END, 0} }; static struct input_dev *dell_wmi_input_dev; static struct key_entry *dell_wmi_get_entry_by_scancode(int code) { struct key_entry *key; for (key = dell_wmi_keymap; key->type != KE_END; key++) if (code == key->code) return key; return NULL; } static struct key_entry *dell_wmi_get_entry_by_keycode(int keycode) { struct key_entry *key; for (key = dell_wmi_keymap; key->type != KE_END; key++) if (key->type == KE_KEY && keycode == key->keycode) return key; return NULL; } static int dell_wmi_getkeycode(struct input_dev *dev, int scancode, int *keycode) { struct key_entry *key = dell_wmi_get_entry_by_scancode(scancode); if (key && key->type == KE_KEY) { *keycode = key->keycode; return 0; } return -EINVAL; } static int dell_wmi_setkeycode(struct input_dev *dev, int scancode, int keycode) { struct key_entry *key; int old_keycode; if (keycode < 0 || keycode > KEY_MAX) return -EINVAL; key = dell_wmi_get_entry_by_scancode(scancode); if (key && key->type == KE_KEY) { old_keycode = key->keycode; key->keycode = keycode; set_bit(keycode, dev->keybit); if (!dell_wmi_get_entry_by_keycode(old_keycode)) clear_bit(old_keycode, dev->keybit); return 0; } return -EINVAL; } static void dell_wmi_notify(u32 value, void *context) { struct acpi_buffer response = { ACPI_ALLOCATE_BUFFER, NULL }; static struct key_entry *key; union acpi_object *obj; wmi_get_event_data(value, &response); obj = (union acpi_object *)response.pointer; if (obj && obj->type == ACPI_TYPE_BUFFER) { int *buffer = (int *)obj->buffer.pointer; printk("dell wmi got code %x\n",value); /* * The upper bytes of the event may contain * additional information, so mask them off for the * scancode lookup */ key = dell_wmi_get_entry_by_scanc ode(buffer[1] & 0xFFFF); if (key) { switch (key->type) { case KE_KEY: input_report_key(dell_wmi_input_dev, key->keycode, 1); input_sync(dell_wmi_input_dev); input_report_key(dell_wmi_input_dev, key->keycode, 0); input_sync(dell_wmi_input_dev); break; case KE_SW: input_report_switch(dell_wmi_input_dev, key->keycode, key->state); input_sync(dell_wmi_input_dev); break; } } else if (buffer[1] & 0xFFFF) printk(KERN_INFO "dell-wmi: Unknown key %x pressed\n", buffer[1] & 0xFFFF); } } static int __init dell_wmi_input_setup(void) { struct key_entry *key; int err; dell_wmi_input_dev = input_allocate_device(); if (!dell_wmi_input_dev) return -ENOMEM; dell_wmi_input_dev->name = "Dell WMI hotkeys"; dell_wmi_input_dev->phys = "wmi/input0"; dell_wmi_input_dev->id.bustype = BUS_HOST; dell_wmi_input_dev->getkeycode = dell_wmi_getkeycode; dell_wmi_input_dev->setkeycode = dell_wmi_setkeycode; for (key = dell_wmi_keymap; key->type != KE_END; key++) { switch (key->type) { case KE_KEY: set_bit(EV_KEY, dell_wmi_input_dev->evbit); set_bit(key->keycode, dell_wmi_input_dev->keybit); break; case KE_SW: set_bit(EV_SW, dell_wmi_input_dev->evbit); set_bit(key->keycode, dell_wmi_input_dev->swbit); break; } } err = input_register_device(dell_wmi_input_dev); if (err) { input_free_device(dell_wmi_input_dev); return err; } return 0; } static int __init dell_wmi_init(void) { int err; if (wmi_has_guid(DELL_EVENT_GUID)) { err = dell_wmi_input_setup(); if (err) return err; err = wmi_install_notify_handler(DELL_EVENT_GUID, dell_wmi_notify, NULL); if (err) { input_unregister_device(dell_wmi_input_dev); printk(KERN_ERR "dell-wmi: Unable to register" " notify handler - %d\n", err); return err; } } else printk(KERN_WARNING "dell-wmi: No known WMI GUID found\n"); return 0; } static void __exit dell_wmi_exit(void) { if (wmi_has_guid(DELL_EVENT_GUID)) { wmi_remove_notify_handler(DELL_EVENT_GUID); input_unregister_device(dell_wmi_input_dev); } } module_init(dell_wmi_init); module_exit(dell_wmi_exit);
-
I'm trying write a script to disable capslock as soon as the button is not actually being held down. I found the following function will return 0 when capslock is toggled off, 1 when it is toggled on, and some other number (varies), when it is physically held down. However, Send("{CAPSLOCK off}") or any variant does not seem to work to actually change the capslock state. Is there any other method to do it? I'd also like to hook the capslock key so I don't have to poll, but I guess that might be awful close to keylogger territory, so I doubt I will get answers on that. *edit* nevermind, got it. #include <WinAPI.au3> $VK_CAPS = 0x14 While 1 $test = _GetCapsLock() If $test = 1 Then ConsoleWrite("Caps On" & @CRLF) ElseIf $test = 0 Then ConsoleWrite("Caps Off" & @CRLF) Else ConsoleWrite("Caps Down" & @CRLF) EndIf Sleep(500) WEnd Func _GetCapsLock() Local $ret $ret = DllCall("user32.dll","long","GetKeyState","long",$VK_CAPS) Return $ret[0] EndFunc
-
Another hook-related problem... Looking for a method to run a script when a particular process is terminated, other than periodically polling for the presence of that process or window. I can't imagine that I'm the first person to want to do this, so either I fail at searching, or it can't be done. Hoping for the former.
-
Windows 7 only supports specific oem logon backgrounds for 4:3 resolutions. This sucks for those of us who happens to end up using multiple aspect ratios. For me, it is because I use a 16:10 tablet pc, which means that quite frequently, it is actually 10:16. the following script overcomes that limitation, with behavior functionally identical to the normal windows 7 logon screen behavior. Thanks to KaFu for overcoming the issue that script execution would be halted while the actual logon screen was displayed... However, I found that it wasn't necessary after all. #cs ---------------------------------------------------------------------------- AutoIt Version: 3.3.6.1 Author: Enneract Script Function: Rotate windows 7 logon screen wallpaper to match any arbitrary resolution changes. #ce ---------------------------------------------------------------------------- #NoTrayIcon $path = "C:\Windows\System32\oobe\info\backgrounds\" $lasth = @DesktopHeight $lastw = @DesktopWidth GUICreate("", 0,0,0,0) GUIRegisterMsg(0x007E, "Display_Changed") while 1 sleep(10) wend Func Display_Changed() If $lasth <> @DesktopHeight OR $lastw <> @DesktopWidth Then FileCopy($path & "background" & @DesktopWidth & "x" & @DesktopHeight & ".jpg", $path & "backgroundDefault.jpg", 1) $lasth = @DesktopHeight $lastw = @DesktopWidth EndIf EndFunc
-
Oooh, I see. _WinEventHook_Proc() is actions to be preformed on the hook. Gotcha. Thanks a bunch, KaFu.
-
I need to delay operation of a script until the lock\welcome screen is no longer present. The script's purpose is to counteract the lack of Win7's ability to use specific logon backgrounds for widescreen resolutions, and applies backgrounds based on whatever arbitrary resolution the desktop is set to, using WM_DISPLAYCHANGE to notice when the change happens. However, none of this seems to work if the logon\lock\welcome screen is up when the display change takes place, so I need to detect when that happens and poll the desktop resolution when the screen is exited. #NoTrayIcon $path = "C:\Windows\System32\oobe\info\backgrounds\" $last = @DesktopHeight Func On_Start() GUICreate("", 0,0,0,0) GUIRegisterMsg(0x007E, "Display_Changed") Do_Copy() EndFunc Func Do_Copy() If NOT FileCopy($path & "background" & @DesktopWidth & "x" & @DesktopHeight & ".jpg", $path & "backgroundDefault.jpg", 1) Then SoundPlay(@WindowsDir & "\media\Windows Error.wav",1) EndIf EndFunc Func Display_Changed() If $last <> @DesktopHeight Then Do_Copy() $last = @DesktopHeight EndIf EndFunc On_Start() While 0 < 1 WEnd
-
I don't understand that code, at all. I can copy and paste with the best of them, but I don't even see how that code interfaces with anything? I see the hook, but I don't understand what triggers it, nor how I can reference it. Can you shed some light, or point me in the direction of some reading material?
-
I hate to start multiple topics, but this is far enough afield from my other to warrant it, I think. I'm trying to find a way to detect the presence of the ctrl-alt-del lock screen, preferably in a way that doesn't require polling, so that I can delay components of script execution until the screen is no longer present. I've been searching for the past two hours or so, with only a reference to looking for the presence of a window titled "Program Manager" to detect if the screen is not present, but this doesn't seem to do anything. Alternatively, a way to register WM_DISPLAYCHANGE and preform file copy operations while the lock screen is present would also work very well.
-
And, of course, cursory investigation yields fruit. GUIRegisterMsg($WM_DiSPLAYCHANGE, "Do_Something") this would work, right? And is there any reason why I shouldn't use GUICreate("", 0,0,0,0) since GUIRegisterMsg requires a gui element to recieve the notification?
-
I have a script which polls the desktop resolution every 30 seconds looking for a change to a particular resolution. Is there a way to use autoit to hook the resolution change system event, so that this script becomes more efficient and responds instantly to the change? I'm not finding anything specific to this on search, if someone could point me in the right direction?
-
I'm trying to capture the scancodes (or, for that matter, otherwise detect the key presses) off my tabletpc's bezel buttons for use in a script to hijack the 'rotate screen' button for another purpose (I have a dell latitude xt2, if anyone has specific info). and... I'm not finding anything. every method I've tried of capturing arbitrary key presses doesn't even notice the bezel buttons. does anyone have any ideas?
-
Disabling mouse, using scroll lock
enneract replied to enneract's topic in AutoIt General Help and Support
Yea... the gaming thing is why I added the toggle. I don't game very often on my tablet, though. That being said, my first version had no toggle, which made me consider that this might be an interesting solution to make gaming on school\company\similar machines impossibly annoying. -
Disabling mouse, using scroll lock
enneract replied to enneract's topic in AutoIt General Help and Support
Ayup. Awesome link, thanks, solved my issues. #include <WinAPI.au3> #include <WindowsConstants.au3> #include <StructureConstants.au3> #include <misc.au3> #include <MouseSetOnEvent_UDF.au3> Global $hHook, $hStub_KeyProc Global $latest = 0 Global Const $VK_SCROLL = 0x91, $timeout = 300 _Main() Func _Main() OnAutoItExitRegister("Cleanup") $hStub_KeyProc = DllCallbackRegister("_KeyProc", "long", "int;wparam;lparam") $hmod = _WinAPI_GetModuleHandle(0) $hHook = _WinAPI_SetWindowsHookEx($WH_KEYBOARD_LL, DllCallbackGetPtr($hStub_KeyProc), $hmod) While 1 If TimerDiff($latest) > $timeout OR _GetScrollLock() = 0 Then _BlockMouseInput(1) EndIf WEnd EndFunc ;==>_Main Func _BlockMouseInput($iOpt=0) If $iOpt = 0 Then _MouseSetOnEvent($MOUSE_PRIMARYUP_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_PRIMARYDOWN_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_SECONDARYUP_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_SECONDARYDOWN_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_MOVE_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_SECONDARYDBLCLK_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_WHEELDOWN_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_WHEELUP_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_WHEELDBLCLK_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_WHEELSCROLL_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_XBUTTONDOWN_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_XBUTTONUP_EVENT, "__Dummy") _MouseSetOnEvent($MOUSE_XBUTTONDBLCLK_EVENT, "__Dummy") Else _MouseSetOnEvent($MOUSE_PRIMARYUP_EVENT) _MouseSetOnEvent($MOUSE_PRIMARYDOWN_EVENT) _MouseSetOnEvent($MOUSE_SECONDARYUP_EVENT) _MouseSetOnEvent($MOUSE_SECONDARYDOWN_EVENT) _MouseSetOnEvent($MOUSE_MOVE_EVENT) _MouseSetOnEvent($MOUSE_SECONDARYDBLCLK_EVENT) _MouseSetOnEvent($MOUSE_WHEELDOWN_EVENT) _MouseSetOnEvent($MOUSE_WHEELUP_EVENT) _MouseSetOnEvent($MOUSE_WHEELDBLCLK_EVENT) _MouseSetOnEvent($MOUSE_WHEELSCROLL_EVENT) _MouseSetOnEvent($MOUSE_XBUTTONDOWN_EVENT) _MouseSetOnEvent($MOUSE_XBUTTONUP_EVENT) _MouseSetOnEvent($MOUSE_XBUTTONDBLCLK_EVENT) EndIf EndFunc ;==>_BlockMouseClicksInput Func _GetScrollLock() Local $ret $ret = DllCall("user32.dll","long","GetKeyState","long",$VK_SCROLL) Return $ret[0] EndFunc Func EvaluateKey() If _GetScrollLock() = 1 Then _BlockMouseInput(0) $latest = TimerInit() EndIf EndFunc ;==>EvaluateKey Func _KeyProc($nCode, $wParam, $lParam) Local $tKEYHOOKS $tKEYHOOKS = DllStructCreate($tagKBDLLHOOKSTRUCT, $lParam) If $nCode < 0 Then Return _WinAPI_CallNextHookEx($hHook, $nCode, $wParam, $lParam) Else EvaluateKey() EndIf Return _WinAPI_CallNextHookEx($hHook, $nCode, $wParam, $lParam) EndFunc ;==>_KeyProc Func Cleanup() _WinAPI_UnhookWindowsHookEx($hHook) DllCallbackFree($hStub_KeyProc) EndFunc ;==>Cleanup It is ugly but it works. Scroll lock toggles it on and off. You need the UDF include linked in the quoted post. -
I'm working on porting a small script written for AHK, designed to disable mouse input for a short time after any key is pressed. (I brush my trackpad on my laptop quite frequently while typing, very annoying). I'm using the example from the documentation _WinAPI_SetWindowsHookEX() as a skeleton, and I have it mostly working... except... 1) the only way of disabling the mouse I've found is _MouseTrap(), but I can't figure out how to use this to trap the mouse in a 1 pixel region where it is. 2) I haven't figured out how to disable mouseclicks, 3) my code for using scroll lock as a toggle doesn't seem to work, and I'm lost as to why, 4) I just thought of this, so I haven't actually looked for one yet, but is there a way to detect scroll lock toggle state? Below is my code (well, only some mine!). If anyone has input as to how to solve the problems listed and are bored\unoccupied enough to share it, I would be appreciative! #AutoIt3Wrapper_Au3Check_Parameters=-d -w 1 -w 2 -w 3 -w 4 -w 5 -w 6 #include <WinAPI.au3> #include <WindowsConstants.au3> #include <StructureConstants.au3> #include <misc.au3> Opt('MustDeclareVars', 1) Global $hHook, $hStub_KeyProc, $buffer = "" Global $latest = 0, $on = 0 _Main() Func _Main() OnAutoItExitRegister("Cleanup") Local $hmod $hStub_KeyProc = DllCallbackRegister("_KeyProc", "long", "int;wparam;lparam") $hmod = _WinAPI_GetModuleHandle(0) $hHook = _WinAPI_SetWindowsHookEx($WH_KEYBOARD_LL, DllCallbackGetPtr($hStub_KeyProc), $hmod) Run("Notepad") WinWait("Untitled -") WinActivate("Untitled -") While 1 If TimerDiff($latest) > 500 Then _MouseTrap () EndIf WEnd EndFunc ;==>_Main Func EvaluateKey($keycode) If ($keycode = 145) Then ; Scroll Lock ConsoleWrite("Scroll LOCK! -" & $keycode & "-" & $on) If $on = 0 Then $on = 1 If $on = 1 Then $on = 0 ElseIf $on = 1 Then _MouseTrap(0, 0) $latest = TimerInit() EndIf EndFunc ;==>EvaluateKey ;=========================================================== ; callback function ;=========================================================== Func _KeyProc($nCode, $wParam, $lParam) Local $tKEYHOOKS $tKEYHOOKS = DllStructCreate($tagKBDLLHOOKSTRUCT, $lParam) If $nCode < 0 Then Return _WinAPI_CallNextHookEx($hHook, $nCode, $wParam, $lParam) Else EvaluateKey(DllStructGetData($tKEYHOOKS, "vkCode")) EndIf Return _WinAPI_CallNextHookEx($hHook, $nCode, $wParam, $lParam) EndFunc ;==>_KeyProc Func Cleanup() _WinAPI_UnhookWindowsHookEx($hHook) DllCallbackFree($hStub_KeyProc) EndFunc ;==>Cleanup
-
I don't see any other way to attempt this. (Although, using ABM_QUERYPOS right after setting the data, but before ABM_SETPOS seems pointless, as ABM_QUERYPOS returns info in that same struct... switching it doesn't help, though.) I don't think it is doable through this API. I think I figured out a method by writing registry values and forcing explorer to reload. Meh. Unless there is a way to manipulate the settings window while it is 'hidden'?
-
DllCall("Shell32.dll", "int", "SHAppBarMessage", "int", $ABM_GETTASKBARPOS, "ptr", $ptAPPBARDATA) Your DLLCall() was wrong, didn't have enough information. That returns real values. However, using that same code and just setting the values that it should be on the right side of the screen, and switching to $ABM_SETPOS, results in nothing at all. I'm at a loss...
-
Global Const $ABM_SETPOS = 0x00000003 GLOBAL CONST $ABE_LEFT = 0x0 GLOBAL CONST $ABE_TOP = 0x1 GLOBAL CONST $ABE_RIGHT = 0x2 GLOBAL CONST $ABE_BOTTOM = 0x3 $hwnd = HWnd(WinGetHandle("[CLASS:Shell_TrayWnd]")) $AppBarData = DllStructCreate("dword;hwnd;uint;uint;int[4];int") DllStructSetData($AppBarData,1,DllStructGetSize($AppBarData)) DllStructSetData($AppBarData,2,$hwnd) DllStructSetData($AppBarData,4,$ABE_RIGHT) DllCall("shell32.dll", "BOOLEAN", "SHAppBarMessage", "int", $ABM_SETPOS, "ptr", DllStructGetPtr($AppBarData)) Exit Is what I have... and if I am understanding the concept correctly, this should work as soon as I figure out how to define the 'rc' array element.
-
Apparently I can't edit. I think I may have it, mostly. I just am having trouble dealing with the element, which is of type 'uint', which specifies which edge the bar is on. However, all the possible values of this are strings.... so I am confused.
-
I think that may be way over my head. Any chance you could point me in the direction for something similar, utilizing such a call & dealing with the pointer it requires?
-
I'm trying to move the taskbar from the bottom to the right side of the screen using autoit. I don't want to simply open the properties menu and use the dropdown box, I'd like it to move without obviously being 'scripted' as such. I also got it to work by using MouseClickDrag(), but I also hate this. the following does not work. WinMove([CLASS:Shell_TrayWnd]", "", 1218, 0, 62, 800) Excuse the hardcoded values, but they are the correct position and size of the window, and I'm just trying to get proof of concept going. Does anyone have any ideas?