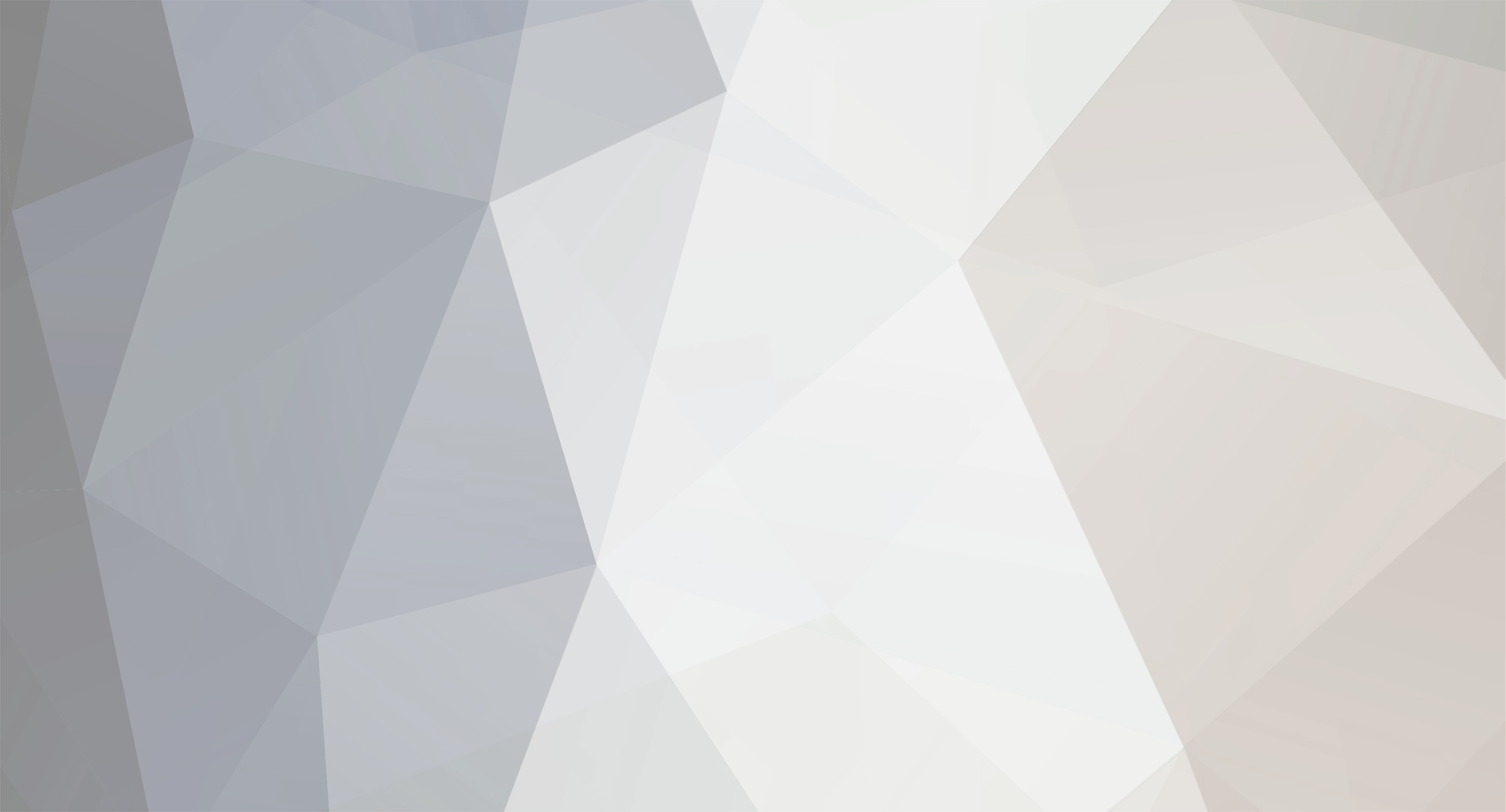
CoderDunn
Active Members-
Posts
336 -
Joined
-
Last visited
Everything posted by CoderDunn
-
Thanks! It took a while to put it all together
-
I'm currently taking an intermediate C++ course at college, and I wrote this file packer/unpacker for an assignment to showcase reading/writing binary files in C++. I figure some one here might have a use for it, so I converted it to a DLL and wrote the wrapper functions so you can use it in AutoIt3. Since I'm really bad at naming things, I'm calling it "Andy's File Packer" or "AFP" for short. I'm also using that as the file extension, but you can use any extension you want. Why use this over FileInstall? Well you can use a variable for the file name, and can extract all the files with a single loop, which is nice. Especially when you have a bunch of files. Unfortunately, it doesn't support compression. However, you could FileInstall() the AFP file and then extract it. Another advantage is that when you open an AFP file, only the embedded file table is loaded in memory. So no matter how big the AFP file is, it shouldn't use much memory (unless of course you pack thousands of files). All of the embedded files can be accessed and unpacked in any order. Example scripts are included to show how to pack and unpack files. It's pretty simple. The C++ source code for the DLL is included, and since I wrote this for a college course, practically every other line is commented. Here is a list of the functions: ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_CreateNew ; Description ...: Creates a new AFP file. Add files with _AFP_AddItem() and then compile the AFP with _AFP_Compile() ; Syntax.........: _AFP_CreateNew($dll, $filePath) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $filePath - Path to the AFP file you wish to create ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - Error creating character array struct to hold the file name ; |2 - DLLCall encountered an error, more specific DLL error code is in @extended ; |3 - DLL function CreateAFPFile() encountered an error, specific error code is in @extended ; | @extended error codes: ; | 0 - Unable to open output file ($filePath) ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_CreateNew($dll, $filePath) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_AddItem ; Description ...: Adds a file to the list of files that will be embedded in an AFP file (created via _AFP_CreateNew()) when ; _AFP_Compile() is called ; Syntax.........: _AFP_AddItem($dll, $descript, $type, $filePath) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $descript - User defined string that describes the to-be-embedded file ; $type - User defined integer that describes the to-be-embedded file's type ; $filePath - Path to the file that will be embedded inside the AFP file ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - $filePath does not exist ; |2 - Error creating character array struct to hold the description ; |3 - Error creating character array struct to hold the file path ; |4 - $type is not an integer ; |5 - DLLCall encountered an error, more specific DLL error code is in @extended ; |6 - DLL function _AFP_AddItem() encountered an error, specific error code is in @extended ; | @extended error codes: ; | 0 - AFP file handle is closed or AFP file was opened via _AFP_Open() ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_AddItem($dll, $descript, $type, $filePath) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_Compile ; Description ...: Takes all the files added via _AFP_AddItem() and packs them into a single AFP file ; Syntax.........: _AFP_Compile($dll, $bool_CloseHandle) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $bool_CloseHandle - Boolean, use True if you want the AFP file handle to be closed when it's done being written ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - $bool_CloseHandle is not a boolean ; |2 - DLLCall encountered an error, more specific DLL error code is in @extended ; |3 - DLL function _AFP_Compile() encountered an error, specific error code is in @extended ; | @extended error codes: ; | 0 - AFP file handle is closed or AFP file was opened via _AFP_Open() ; | -1 - Error while writing the file header ; | -2 - Error while writing the embedded file table ; | -3 - Error while writing the embedded file's data (one of the files added via _AFP_AddItem() doesn't exist, ; or is inaccessible) ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_Compile($dll, $bool_CloseHandle = True) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_Open ; Description ...: Opens an AFP file that was previously created via _AFP_CreateNew() ; Syntax.........: _AFP_Open($dll, $filePath) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $filePath - Path to the AFP file you wish to open ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - Error creating character array struct to hold the file name ; |2 - DLLCall encountered an error, more specific DLL error code is in @extended ; |3 - DLL function OpenAFPFile() encountered an error, specific error code is in @extended ; | @extended error codes: ; | 0 - Unable to open input file ($filePath) ; | -1 - Unknown or corrupted file ; | -2 - Error while reading the file header ; | -3 - Error while reading the embedded file table ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_Open($dll, $filePath) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_GetNumItems ; Description ...: Returns the number of embedded files within the AFP opened via _AFP_Open() ; Syntax.........: _AFP_GetNumItems($dll) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; Return values .: Success - The number of embedded files within the AFP file ; Failure - 0, sets @error and @extended (when needed) ; |1 - DLLCall encountered an error, more specific DLL error code is in @extended ; |2 - DLL function GetNumberOfItems() encountered an error, specific error code is in @extended ; | @extended error codes: ; | -1 - AFP file handle is closed ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_GetNumItems($dll) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_GetItemInfo ; Description ...: Returns an array containing information about an embedded file within an AFP file that was opened via _AFP_Open() ; Syntax.........: _AFP_GetItemInfo($dll, $index) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $index - Index of the embedded file (starting at 0) ; Return values .: Success - A four element array containing the following: ; $returnArr[0] - Description ; $returnArr[1] - Type ; $returnArr[2] - Position of the embedded file within the AFP (starting after the embedded file table's postion) ; $returnArr[3] - Size of the embedded file ; Failure - 0, sets @error and @extended (when needed) ; |1 - Index is out of range or AFP file handle is closed ; |2 - DLL function GetItemInfo() encountered an error ; |3 - Error creating a struct from the returned pointer, DllStructCreate() error code is in @extended ; |4 - Error creating description (char array) struct from pointer, DllStructCreate() error code is in @extended ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_GetItemInfo($dll, $index) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_ExtractFile ; Description ...: Extracts a file from an AFP file that was opened via _AFP_Open() ; Syntax.........: _AFP_ExtractFile($dll, $index, $filePath) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; $index - Index of the file that you want to extract (starting at 0) ; $filePath - The file that it will be extracted to (Note: If file exists, it will be over written) ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - Error creating character array struct to hold the file name ; |2 - $index is not an integer ; |3 - DLLCall encountered an error, more specific DLL error code is in @extended ; |4 - DLL function ExtractFile() encountered an error, specific error code is in @extended ; | @extended error codes: ; | 0 - AFP file handle is closed or AFP file was opened via _AFP_CreateNew() ; | -1 - $index is out of bounds ; | -2 - Error opening output file path ; | -3 - Error reading embedded file data ; | -4 - Error writing embedded file data to output file ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_ExtractFile($dll, $index, $filePath) ; #FUNCTION# ==================================================================================================================== ; Name...........: _AFP_Close ; Description ...: Closes the AFP file handle that is opened after calling either _AFP_CreateNew() or _AFP_Open() ; Syntax.........: _AFP_Close($dll) ; Parameters ....: $dll - Handle to Andy's File Packer dll via DLLOpen() ; Return values .: Success - 1 ; Failure - 0, sets @error and @extended (when needed) ; |1 - DLLCall encountered an error, more specific DLL error code is in @extended ; Author ........: Andrew Dunn (forums: CoderDunn) ; =============================================================================================================================== Func _AFP_Close($dll) And for those interested, here is the binary format specifications for an AFP file The AFP Binary File Format Note for readability, I placed each separate piece on it's own line. However, in a real AFP file, they would all be on the same line with no delimiters. ======= Start of AFP File ======= [Null terminated string header, "Andy's File Packer 2.0"] [4 byte integer which holds the number of embedded files] ~ Begin Embedded File Table ~ [Embedded File 1 Description, null terminated string] [Embedded File 1 Type, 4 byte integer] [Embedded File 1 Position in AFP file, 4 byte integer] [Embedded File 1 Size, 4 byte integer] ... [Embedded File n Description, null terminated string] [Embedded File n Type, 4 byte integer] [Embedded File n Position in AFP file, 4 byte integer] [Embedded File n Size, 4 byte integer] ~ End Embedded File Table ~ ~ Start Embedded File Raw Data ~ [Embedded File 1 Raw Data] ... [Embedded File n Raw Data] ~ End Embedded File Raw Data ~ ======= End of AFP File ======= If you have any questions or run into any problems, let me know Download Here
-
I figured it out, thanks! I changed the function to use a stdcall and a .def file to export the function, and now it works int _stdcall TestFunc(int x, int y) { return x + y; }
-
I'm taking an intermediate C++ course in college, and I'm wondering how to write a DLL that can be called within AutoIt3. I tried to make a very simple dll with a function that adds two integers, however when I try to call it from within AutoIt, the script either locks up or throws an error 3 ("function" not found in the DLL file). I was able to compile an AutoIt plugin that worked fine, but I can't get a standard DLL to work ... Here is the dll (test dll.cpp): __declspec(dllexport) int TestFunc(int x, int y) { return x + y; } And here is the script: $dll = DllOpen("Test DLL.dll") if (@error) Then FatalError("Unable to open DLL") $result = DllCall($dll, "int", "TestFunc", "int", 3, "int", 4) if (@error) Then FatalError("Error calling DLL: " & @error) MsgBox(0, "Result", $result[0]) Func FatalError($msg) MsgBox(0, "Fatal Error", $msg) Exit EndFunc Any help would be much appreciated. Thanks, Andy
-
Nope just been busy with school. It's my last year of highschool and I have a lot of after school activites, not to mention the massive 'Senior Project' we have to do. I'm not completely sure how do do a few things anyways so Iv'e been reading books I got from the local bookstore Thanks for letting me know, I'm completely rewriting my file transfer system so hopefully it will be fixed. About the screen shot thing ... That's wierd i'll try to re-create the error. Right now I'm looking into adding Lua support so people can make addons. ~ Hallman
-
It's hard to say since I don't have a regular schedule, I just work on it when I can. Here's about how much I've done. Main UI = 70% done Other forms = 40% done Network engine = 95% done Network functions = 15% done I'll try to keep this updated ... CODE === September 11, 2008 === Sorry for no update in a while, first two weeks of school has kept me busy! - Planned out most of the server - Started working on the "File Transfer Service" which moves all file transfers into the same thread independent from the rest of the server. === August 30, 2008 === Going up in the mountains with my friend to ride quads, be back on Monday. School starts on Tuesday >.< === August 28, 2008 - Began to completely re-design the System Info tab so it's more organized, has more information, and takes less time to load === August 27, 2008 === - Added more functions for the Client List View Context Menu - Created a few methods for getting the selected client's info - Added Log off, hibernate, shut down, etc commands - Changed the wording of a few console messages - The IP column in the client list now includes the remote end point in parentheses EX: "192.168.1.100 (65.42.10.96:50431)" === August 26, 2008 === - Split client list view into two lists. One for online users, the other for offline users. - Added a context menu to the client list view with the "Set User Name" item - Ran a few stress tests, and fixed some major bugs causing the server to completely lock up if more than one user connected at the same time. === August 25, 2008 === After playing around with a few different ways to store data, I decided (at least for now) to use the registry to store settings and client information =O I know many people will complain about this but it works very well, and is less likely to have problems or get manually edited. === August 24, 2008 === Huge set back today, I just discovered my XML write method has some major problems. I need to rewrite it or find a better storage format. === August 23, 2008 === Just trying some new things out - Created template for "clients.xml" I plan on using this file to store client data when they are offline. - The client list is now "grouped" - Connected clients are under the "Online" group, disconnected users are under the "Offline" group - I'm in the process of removing all the message boxes, and replacing them with a "Message Log" tab on the main form. - Experimenting with a PCID filter - Experimenting with a "Status" tab that shows the current and total net usage. I'm thinking about adding an individual client net usage monitor. === August 22, 2008 === - Finished most of the network engine - Started the "Skin Editor" form - Added method to invoke functions on the UI thread ~ Hallman
-
lol ... First of all, don't double post. Especially an inconsiderate post like yours. Second, if you want someone to get your point, it's proabably best not to contradict yourself in your first few, incomplete sentences. My "damn program sucks", and yet it's great? Third, before you complain about my FREE open source application, think about how much work it is to create what I have, or just the simple fact that you have not contributed a single thing to this forum. Fourth, If you could read, you would notice quite clearly the title says BETA, which means it's still in development. ~ Hallman
-
You all are going to think I'm crazy but ... I have started to rewrite the server for a 4th time! Why? The previous one was fine but because the way it was set up, adding some of the newer features I wanted was next to impossible without fundamentally changing how the core of it works. What can I expect in the next version? A few of the things I'm working on right now: I finally figured out how to make the server resizable!Skin Editor to make your own skins for the serverA little more organizedThere will be a File Transfer tab with a queue for downloads/uploadsI should be ready with a release in a few weeks as I'm working on it in my free time between fixing up the house and getting it ready for sale. ~ Hallman
-
Hey all sorry for the late reply. Iv'e been really busy lately because my dad has decided he hates the big city and he wants to move to Noth Dakota It sucks because I don't want to leave all my freinds behind We have been fixing up the house and getting it ready to sell. We still have a lot to do. Don't worry! I'll be back with in update in a few weeks. In my free time, iv'e been learning more of C# so I can fix many of the things I have done wrong, for example I never used the lock{ } keyword on objects shared between threads Anyways take care and wish me luck! ~ Hallman
-
Hey guys muttley Just letting you know that I'm leaving on a road trip (I think my dad is crazy with the price of gas and all) and I won't be back until about the 28th. So, unfortunately the next release will have to wait until then. Happy coding! ~ Hallman
-
L|M|TER CD/DVD Burner 1.0.2 - XP Support !
CoderDunn replied to LIMITER's topic in AutoIt Example Scripts
Very nice GUI I could learn a thing or two from you I'll test the burning part later when I buy some CD-RW's. ~ Hallman PS Thank you very much for putting my app in your sig! muttley -
Ok I'll see if I can find a possible cause anyways. And your welcome muttley I'm glad to know that my work isn't all to waste
-
Ok next step: Try running the client as a script and as a compiled script and see if they both don't work.
-
lol Good Luck then! Cool muttley I'm glad it's fixed (I didn't so anything so yay it fixed itself ) Aha well it looks like the client isn't connecting for some reason. On the client machine, can you verify that when you start a new file transfer, there are two clients running in the task manager? I plan on doing a complete re-write of the "File Transfer" code for the next update. Hopefully it will fix this! lol good point. I think I will ... I agree, and I have already started working on it for the next version Your Welcome I'm glad it helped you. I am going to post the latest source code when I get it more stable. Right now I'm just updating to often. ~ Hallman
-
Thanks! I may not be the fastest but at least I try to make quality applications
-
Just for fun, I ran the server and the client on the same machine, then opened the screen viewer and maximized it. This is what happened! It's fun then to move the mouse to the far left and wave it slowly up and down. You get a mouse trail as it moves closer and closer to the middle muttley
-
Version 0.5.4.0 Released The Screen Viewer is now in it's own window and auto refreshes! CODE7/04/2008 === Version 0.5.4.0 === - Removed: Pic Control from the "View Screen" tab - Changed: Clicking "View Screen" now opens a new window titled "Screen Viewer" to view the screenshot - Changed: The "Screen Viewer" is now resizable - Added: Auto-Refresh feature to the "Screen Viewer" - Added: Shrink to Fit option to the "Screen Viewer" - Added: View Screen Quality option to the "Options" window in the "Client Settings" tab - Fixed: The "Screen Viewer" now only updates when it is the active window I just tested the downloader and the uploader and they both still work for me. Do you get any message in the console? Same thing with the second comment. If I close the server while it's maximized, it is still maximized when I bring it back. I'm on vista however, maybe it's an XP only bug? I'll test it later on my XP box when I have time. ~ Hallman
-
Version 0.5.3.0 Released CODE7/04/2008 === Version 0.5.3.0 === - (Hopefully) Fixed: Major bug where packets would be partially received during heavy communication Sorry for the quick update! Please test it and see if you get and error. I think I finally fixed it muttley That's why it's in BETA right? I'll see if I can make a search function ... ~ Hallman
-
Version 0.5.2.0 Released Warning! Because of access restrictions in Vista on the registry, the server now requires administrator rights to run CODE7/03/2008 === Version 0.5.2.0 === - Added: Controls to the Options form ---- Run on system startup ---- Start Hidden ---- Timestamp Console messaged ---- Port number ---- Listen IP - Added: Functions to read/write settings settings to and from the XML settings file - Changed: Server no longer refuses to start if settings file is missing. - Changed: Server (unfortunatly) now requires administrator rights so it can access the registry - Changed: If the server is blocked by your fire wall, it will retry 3 times before displaying the "Error creating listening socket" error - Changed: The "Display Message Box" command's title and icon - Fixed: The "Display Message Box" command no longer pauses the client until the message box is closed 7/02/2008 === Version 0.5.1.0 === - Added: Options Dialog, "Scheduled Tasks" and "Plugins" tabs. - Fixed: The server's main form no longer flashes on the screen when ran with the /silent command parameter - Fixed: Typo (thanks JamesB) - Changed: The settings file is now in in the XML format - Changed: The Disconnect and Shutdown buttons now have better titles Thanks, I think I know how to finally fix it now based on that error. It looks like the server is capturing a packet before it is completely received, therefore getting an array out of bounds error after I split it and try to use it's data! Ugh the joys of learning a new language ... And about the shut down thing, I guess there is something in C# that I need to do to have the application close when the system shuts down. I figured it would close automatically but I guess not. Thanks for the info, I'll look into it. ~ Hallman
-
Version 0.5.0.0 released (Yes there is finally a version number) CODE7/01/2008 === Version 0.5.0.0 === - Added: Single instance check for server (was harder than I thought xD) - Changed: "Are you sure?" message box titles to "Radical 2 Server" - Changed: File transfer connections no longer appear in the client list - Changed: File transfers no longer use the standard notifications in the console I'll try to figure an auto refresh out. I will see if I can make a form like the Fire Fox download window. Disconnecting was more for testing purposes at the time. I will change it to "Restart Client" or something Done. I could, but I want Shell Execute to work for things other than just URL's Done. The "Shutdown" button under the client list does this. A client turns red when it's "busy". It's meant to prevent you from sending the client packets while it's in a block of code (like uploading a screenshot). The server pings the clients once every 5 seconds, so that's why it is turning red. Do you think the client turning red is a bad thing or a good thing? ~ Hallman
-
Both xD (duo boot) But lately I've been using Vista more (shudders) since that's what I have Visual Studio 2008 installed on
-
Minor update Fixed the pic control in the View Screen tabChanged "Open Link in Internet Explorer" to "Shell Execute". The downside: to open a link in the web browser, you now have to type the entire URL. Example: "http://www.google.com" instead of "google.com"Moved the "Refresh" buttons outside of the scroll box for the System Info and View Screen tabs. I don't know why I didn't do this to begin with ...Added baloon tip notifications for connecting and disconnecting Suggestions for Hallman's project Options menu item (Server) Where to write the Server port (Registry or Ini)Server startup settings (Maximized, Minimized, Hidden, etc. [if Possible])Allow the screenshot to be a .png or others, to transfer fasterIt shall be done! As soon as I make the options dialog and figure out how to write settings Fix the flash bug when starting up with: "/Silent" I think I know how to fix this. There is no "Start Hidden" option for the form, but there is a "Start Minimized" and a "Don't show in task bar" option Remove the Uploading/Downloading connection establishment (Where it will flash on the Server side) This one will have to wait a bit, since I'm not completely sure how I'm going to make it work yet.Add a plugin system Same as last commentComplete control of a Clients system I'm afraid I can't guarantee this one, but I will see what I can do.Real-Time video from a Client Sorry, but most likely this won't happen. Unless someone knows how to capture video using AutoIt?Set the Client and/or Server to run as a Service This will have to wait until I make an installerInstaller for Server and/or Client Not sure when I'll get to this, but hopefully soonFix the small bug with the Screenshot, no bottom scroll bar, and doesn't scroll properly Done.Allow the Client program to write settings to the Registry Later, keep in mind this is still just the beta Add more options to the Client side, like BlockInput() ShellExecute(), etc. Will be done soon (hopefully)Set the Client to have _SingleTon() (_CheckMutex() ), and #NoTrayIcon Will be done soon (hopefully)Allow Server to be a resizable box As you can see, the server in fact does have a sizable border. But, for some reason I can't get to work Remembering last position/size when program started Once I get the settings stuff doneWhen a Client connects, display a tooltip Done.When a Client disconnects, display a tooltip Done.Shellexecute URL opens URL in user Default Browser in new tab if im not mistaking, Why not to use this instead Not sure why I did it the way I did. I will change it by the next updateAfter you double click on the Blue Drag window bar it will resize gui to Full Desktop size but you cant change its size after you double click the drag window bar again afterwards. I have no idea why it does this. I will look into it thanks. For now, the Maximize button works like it should.The screen Capture option is cool, but since its opening the picture inside the GUI, its really pointless, since it cuts 70% of the picture, so it would be smarter to open it in new GUI. Even cooler would be an option to be able to AUTO screen capture every 3 seconds for example or user can set the time to wait until next auto capture, but I guess new GUI is required to make the optimal use of it. I understand, and it will be done but later. Right now I'm trying to get everything done simple, then will improve them later. If you maximize the server window, and drag the slider separating the client list and the right panel, the screenshot tab will hopefully be big enough to where you don't have to scroll so much.Can only download & upload 1 file at the time? ( Why not to make a Queue option ) You should be able to do multiple downloads and uploads, because each one is on it's own connection, therefore on it's own thread. Do you get a message when trying to do more than one?IT downloads a file from Client Machine auto to my Desktop, let user choose where to save files to. I understand, and it will be done but later. Right now I'm trying to get everything done simple, then will improve them later. But it should be done pretty soon now that Iv'e figured out how to use a File Save Dialog in C#Watch-Over Possibly a "Keylogger" (Full day, Save) Now that AutoIt has removed the limit on hotkeys, it shouldn't be to hard. The question is SHOULD I do it. I don't want my application to be used as a snooping tool for people's passwords.Full day Video record (Full day, Save) I was thinking, record all day, (Unless stopped), have it saved on the Clients computer, and then 'Act' a download, and download it the Server computer to watch. Sorry, but this most likely wont happen. First of all, a 24 hour video at desktop resolution would be HUGE. Not to mention I don't now how to capture a video anyways. But, it did give me an idea. I was thinking an option to have the client capture a screenshot every X amount of seconds and organize them in folders corresponding the the current date and the file name corresponding to the current timeLove this program more and more and Thank Hallman over and over! Thank you (and goldenix) for your suggestions and input It auto-refreshes. Pings auto-refresh every 5 seconds. It shall be done! (Well actually it's already done. "Open link in internet explorer" is now "shell execute") ~ Hallman
-
lol ... Yeah I will add those things to the options My sig is pretty simple. The image is hosted on http://imageshack.us/ [color="#0000FF"][size=2]If you are a System Administrator or just using it for fun, try it out![/size][/color] [url="http://www.autoitscript.com/forum/index.php?showtopic=72298"][img]http://img204.imageshack.us/img204/5327/yellow12rq4.png[/img][/url] [size=3][url="http://www.autoitscript.com/forum/index.php?showtopic=72298"]Radical 2[/url] [color="#FF0000"][i]Remote Administration over the TCP Protocol[/i][/color][/size] [size=2]You know you want to click the yellow button;)[/size] I know the /silent command is kinda wierd. It took me an hour to figure out how to just do that lol ... There is no option in the C# form designer to start a form hidden, so I have to hide it after it apears. And since it's event driven, I have to wait for the WM_PAINT message to fire the event to hide the form. I think I have a better way to do it though, so when I figure it out I'll post it. The icon thing is caused by the same reason. I can't update it until after the form is created. Yes, it's supposed to be gray when it starts up. Gray means no clients are connected. Once a user connects, it will turn yellow. And finally, about the screenshot thing: I just recently switched to using Visual Studio on Vista because on XP 64 bit some things didn't work right. For example, the find dialog (ctrl + F) crashed Visual Studio everytime I used it. However, the transition didn't go smoothly When I opened the project file on vista it moved all the controls around and was a big pain in the arse to fix. I thought I had gotten them all back in place but I guess not. Will update later when I get home. Thank you for the input ~ Hallman