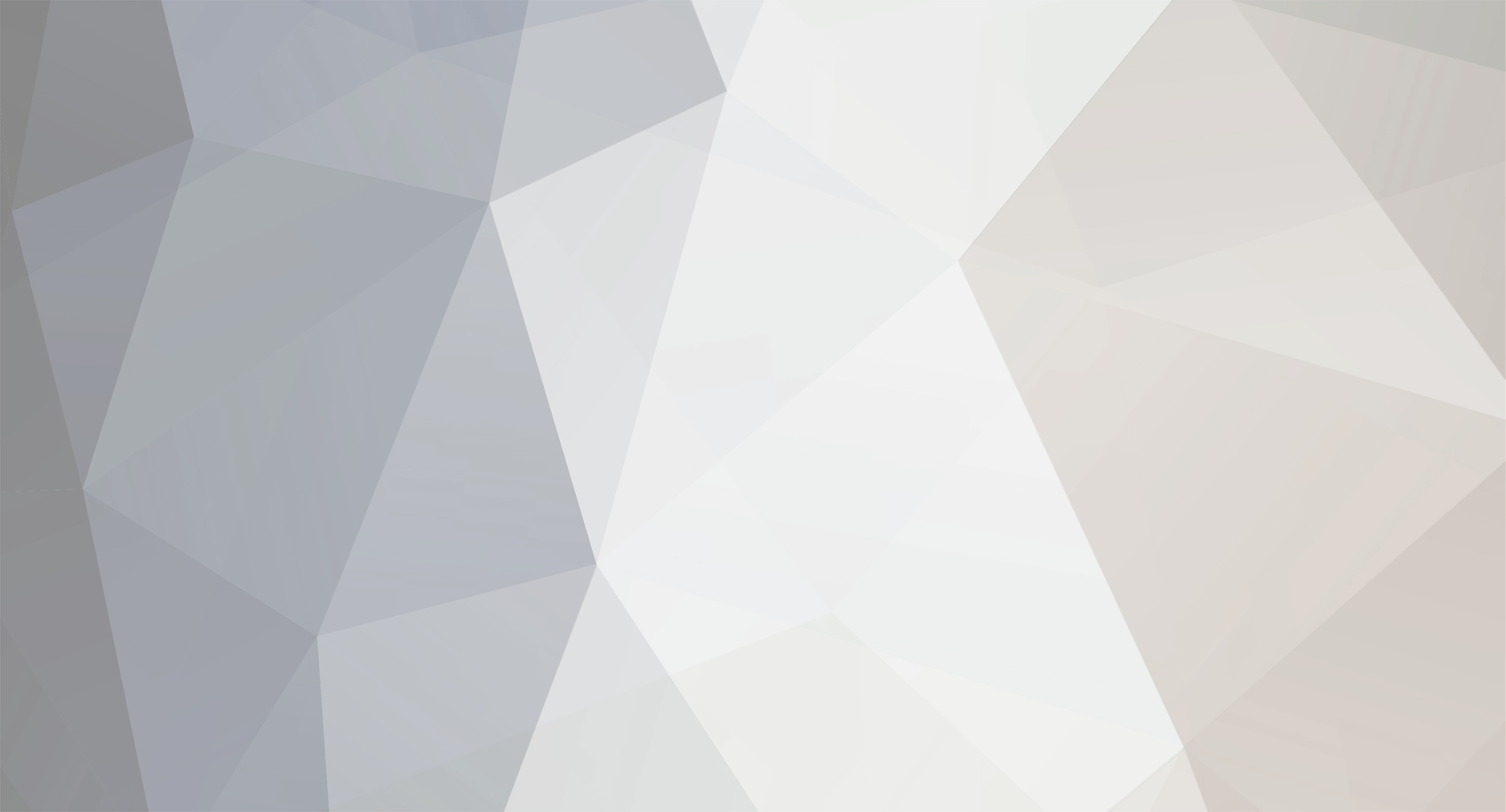
ZoSTeR
Members-
Posts
16 -
Joined
-
Last visited
ZoSTeR's Achievements

Seeker (1/7)
0
Reputation
-
This only works with the Homecinema branch of Media Player Classic (link). Refer to the MpcApi.h file in the SVN for details and updates (link log) The remote control API works by sending WM_COPYDATA messages in both directions. The source should be pretty self explanatory. #include <ButtonConstants.au3> #include <EditConstants.au3> #include <GUIConstantsEx.au3> #include <WindowsConstants.au3> #include <SendMessage.au3> #Include <WinAPI.au3> ; FROM MpcApi.h : ; ;~ // This file define commands used for "Media Player Classic - Homecinema" API. To send commands ;~ // to mpc-hc, and receive playback notifications, first launch process with the /slave command line ;~ // argument follow by an HWnd handle use to receive notification : ;~ // ;~ // ..\bin\mplayerc /slave 125421 ;~ // ;~ // After startup, mpc-hc send a WM_COPYDATA message to host with COPYDATASTRUCT struct filled with : ;~ // - dwData : CMD_CONNECT ;~ // - lpData : Unicode string containing mpc-hc main window Handle ;~ // ;~ // To pilot mpc-hc, send WM_COPYDATA messages to Hwnd provided on connection. All messages should be ;~ // formatted as Unicode strings. For commands or notifications with multiple parameters, values are ;~ // separated by | ;~ // If a string contains a |, it will be escaped with a \ so a \| is not a separator ;~ // ;~ // Ex : When a file is openned, mpc-hc send to host the "now playing" notification : ;~ // - dwData : CMD_NOWPLAYING ;~ // - lpData : title|author|description|filename|duration ;~ #pragma once ;~ typedef enum MPC_LOADSTATE ;~ { ;~ MLS_CLOSED, ;~ MLS_LOADING, ;~ MLS_LOADED, ;~ MLS_CLOSING ;~ }; ;~ typedef enum MPC_PLAYSTATE ;~ { ;~ PS_PLAY = 0, ;~ PS_PAUSE = 1, ;~ PS_STOP = 2, ;~ PS_UNUSED = 3 ;~ }; ;==== Commands from MPC to host ; // Send after connection ; // Par 1 : MPC window handle (command should be send to this HWnd) Global Const $CMD_CONNECT = 0x50000000 ; // Send when opening or closing file ; // Par 1 : current state (see MPC_LOADSTATE enum) Global Const $CMD_STATE = 0x50000001 ; // Send when playing, pausing or closing file ; // Par 1 : current play mode (see MPC_PLAYSTATE enum) Global Const $CMD_PLAYMODE = 0x50000002 ; // Send after opening a new file ; // Par 1 : title ; // Par 2 : author ; // Par 3 : description ; // Par 4 : complete filename (path included) ; // Par 5 : duration in seconds Global Const $CMD_NOWPLAYING = 0x50000003 ; // List of subtitle tracks ; // Par 1 : Subtitle track name 0 ; // Par 2 : Subtitle track name 1 ; // ... ; // Par n : Active subtitle track, -1 if subtitles disabled ; // ; // if no subtitle track present, returns -1 ; // if no file loaded, returns -2 Global Const $CMD_LISTSUBTITLETRACKS = 0x50000004 ; // List of audio tracks ; // Par 1 : Audio track name 0 ; // Par 2 : Audio track name 1 ; // ... ; // Par n : Active audio track ; // ; // if no audio track present, returns -1 ; // if no file loaded, returns -2 Global Const $CMD_LISTAUDIOTRACKS = 0x50000005 ; // List of files in the playlist ; // Par 1 : file path 0 ; // Par 2 : file path 1 ; // ... ; // Par n : active file, -1 if no active file Global Const $CMD_PLAYLIST = 0x50000006 ; ==================================================================== ; ==== Commands from host to MPC ; // Open new file ; // Par 1 : file path Global Const $CMD_OPENFILE = 2684354560 ; 0xA0000000 ; // Stop playback, but keep file / playlist Global Const $CMD_STOP = 2684354561 ; 0xA0000001 ; // Stop playback and close file / playlist Global Const $CMD_CLOSEFILE = 2684354562 ; 0xA0000002 ; // Pause or restart playback Global Const $CMD_PLAYPAUSE = 0xA0000003 ; // Add a new file to playlist (did not start playing) ; // Par 1 : file path Global Const $CMD_ADDTOPLAYLIST = 0xA0001000 ; // Remove all files from playlist Global Const $CMD_CLEARPLAYLIST =0xA0001001 ; // Start playing playlist Global Const $CMD_STARTPLAYLIST = 0xA0001002 ; CMD_REMOVEFROMPLAYLIST = 0xA0001003, // TODO ; // Cue current file to specific position ; // Par 1 : new position in seconds Global Const $CMD_SETPOSITION = 0xA0002000 ; // Set the audio delay ; // Par 1 : new audio delay in ms Global Const $CMD_SETAUDIODELAY = 0xA0002001 ; // Set the subtitle delay ; // Par 1 : new subtitle delay in ms Global Const $CMD_SETSUBTITLEDELAY = 0xA0002002 ; // Set the active file in the playlist ; // Par 1 : index of the active file, -1 for no file selected ; // DOESN'T WORK Global Const $CMD_SETINDEXPLAYLIST = 0xA0002003 ; // Set the audio track ; // Par 1 : index of the audio track Global Const $CMD_SETAUDIOTRACK = 0xA0002004 ; // Set the subtitle track ; // Par 1 : index of the subtitle track, -1 for disabling subtitles Global Const $CMD_SETSUBTITLETRACK = 0xA0002005 ; // Ask for a list of the subtitles tracks of the file ; // return a CMD_LISTSUBTITLETRACKS Global Const $CMD_GETSUBTITLETRACKS = 0xA0003000 ; // Ask for a list of the audio tracks of the file ; // return a CMD_LISTAUDIOTRACKS Global Const $CMD_GETAUDIOTRACKS = 0xA0003001 ; // Ask for the properties of the current loaded file ; // return a CMD_NOWPLAYING Global Const $CMD_GETNOWPLAYING = 0xA0003002 ; // Ask for the current playlist ; // return a CMD_PLAYLIST Global Const $CMD_GETPLAYLIST = 0xA0003003 ;== End MPC Commands Global $gs_messages Global $ghnd_MPC_handle GUIRegisterMsg($WM_COPYDATA, "__Msg_WM_COPYDATA") ; GUI start $Form1 = GUICreate("MPC-HC remote demo", 608, 408, 180, 120) $Edit1 = GUICtrlCreateEdit("", 16, 16, 577, 321) $Button_open = GUICtrlCreateButton("Open File", 24, 368, 140, 28, 0) $Button_play = GUICtrlCreateButton("Play / Pause", 224, 368, 140, 28, 0) $Button_stop = GUICtrlCreateButton("Stop", 424, 368, 140, 28, 0) GUISetState(@SW_SHOW) ; GUI end ; Run MPC-HC with our GUI form handle $s_formHandle = Dec(StringMid(String($Form1),3)) $pid_MPC = Run("mplayerc.exe /slave " & $s_formHandle) If $pid_MPC = 0 Then MsgBox(0,"Error","Could not run Mplayerc.exe (Path?)") Exit EndIf ;GUI loop While 1 $nMsg = GUIGetMsg() Switch $nMsg Case $GUI_EVENT_CLOSE Exit Case $Button_open $s_FileToOpen = FileOpenDialog("Open Media File","C:","Media files (*.*)") __MPC_send_message($ghnd_MPC_handle, $CMD_OPENFILE, $s_FileToOpen) Case $Button_play __MPC_send_message($ghnd_MPC_handle, $CMD_PLAYPAUSE, "") Case $Button_stop __MPC_send_message($ghnd_MPC_handle, $CMD_STOP, "") EndSwitch WEnd Func __MPC_send_message($prm_hnd_MPC, $prm_MPC_Command,$prm_MPC_parameter) Local $StructDef_COPYDATA Local $st_COPYDATA Local $p_COPYDATA Local $st_CDString Local $p_CDString Local $i_StringSize $StructDef_COPYDATA = "dword dwData;dword cbData;ptr lpData"; 32 bit command; length of string; pointer to string $st_COPYDATA = DllStructCreate($StructDef_COPYDATA) ; create the message struct $i_StringSize = StringLen($prm_MPC_parameter) + 1 ; +1 for null termination $st_CDString = DllStructCreate("wchar var1[" & $i_StringSize & "]") ; the array to hold the unicode string we are sending DllStructSetData($st_CDString,1,$prm_MPC_parameter) ; set parameter string DllStructSetData($st_CDString,1,0,$i_StringSize) ; null termination $p_CDString = DllStructGetPtr($st_CDString) ; the pointer to the string DllStructSetData($st_COPYDATA, "dwData", $prm_MPC_Command) ; dwData 32 bit command DllStructSetData($st_COPYDATA, "cbData", $i_StringSize * 2) ; size of string * 2 for unicode DllStructSetData($st_COPYDATA, "lpData", $p_CDString) ; lpData pointer to data $p_COPYDATA = DllStructGetPtr($st_COPYDATA) ; pointer to COPYDATA struct _SendMessage($prm_hnd_MPC,$WM_COPYDATA,0,$p_COPYDATA) $st_COPYDATA = 0 ; free the struct $st_CDString = 0 ; free the struct EndFunc Func __Msg_WM_COPYDATA($hWnd, $Msg, $wParam, $lParam) ; Receives WM_COPYDATA message from MPC ; $LParam = pointer to a COPYDATA struct Local $StructDef_COPYDATA Local $st_COPYDATA Local $StructDef_DataString Local $st_DataString Local $s_DataString Local $s_dwData, $s_cbData, $s_lpData $StructDef_COPYDATA = "dword dwData;dword cbData;ptr lpData" $StructDef_DataString = "char DataString" $st_COPYDATA = DllStructCreate($StructDef_COPYDATA, $LParam) $s_dwData = DllStructGetData($st_COPYDATA,"dwData") ; 32bit MPC command $s_cbData = DllStructGetData($st_COPYDATA,"cbData") ; length of DataString $s_lpData = DllStructGetData($st_COPYDATA,"lpData") ; pointer to DataString $StructDef_DataString = "wchar DataString[" & int($s_cbData) & "]" ;unicode string with length cbData $st_DataString = DllStructCreate($StructDef_DataString,$s_lpData) $s_DataString = DllStructGetData($st_DataString,"DataString") ; If we receive a 0x50000000 CMD_CONNECT we retrieve the MPC handle If $s_dwData = 1342177280 Then $ghnd_MPC_handle = $s_DataString EndIf $gs_messages = __MPC_Command_to_Text($s_dwData) & @CRLF & "Data: " & $s_DataString & @CRLF & $gs_messages GUICtrlSetData($Edit1, $gs_messages) EndFunc Func __MPC_Command_to_Text($prm_MPC_Command) ; Converts an MPC command code to plain text; accepts the decimal value of the command Switch $prm_MPC_Command Case 1342177280 ;0x50000000 $s_return_msg = "CMD_CONNECT" Case 1342177281 ;0x50000000 $s_return_msg = "CMD_STATE" Case 1342177282 ;0x50000002 $s_return_msg = "CMD_PLAYMODE" Case 1342177283 ;0x50000003 $s_return_msg = "CMD_NOWPLAYING" Case 1342177284 ;0x50000004 $s_return_msg = "CMD_LISTSUBTITLETRACKS" Case 1342177285 ;0x50000005 $s_return_msg = "CMD_LISTAUDIOTRACKS" Case 1342177286 ;0x50000006 $s_return_msg = "CMD_PLAYLIST" Case Else $s_return_msg = "CMD_NO_or_UNKNOWN_CMD" EndSwitch Return $s_return_msg EndFunc
-
You could use two timer variables: $timer1 = TimerInit() $timer2 = TimerInit() While 1 sleep(50) $diff1 = TimerDiff($timer1) If $diff1 >= 2000 Then ConsoleWrite("1: " & $diff1 & @CRLF) $timer1 = TimerInit() EndIf $diff2 = TimerDiff($timer2) If $diff2 >= 500 Then ConsoleWrite("2: " & $diff2 & @CRLF) $timer2 = TimerInit() EndIf WEnd And please use the [ AutoIt ] tags or at least a different color. I nearly went blind
-
In case you run one script from the other you could also use EnvSet / EnvGet, STDIO functions, command line parameters or even TCP/IP communication.
-
With the new feature to send binary streams via STDIO you can do a whole bunch of things with the VLC Player (link). Sample: $f_MediaFile = FileOpen("C:\somemovie.avi",0+16) $p_VLC = Run("vlc.exe -","",@SW_MAXIMIZE,1) ; the "-" as parameter tells VLC to take STDIO as input While 1 $buffer = FileRead($f_MediaFile,1024) If @error = -1 Then ExitLoop StdinWrite($p_VLC,$buffer) Wend FileClose($f_MediaFile) Instead of streaming from a file you can send the TCP buffer to VLC. Just make sure you use the latest Autoit version (v3.2.12.0).
-
WeaponX created a whole bunch: link
-
Not to discourage you from your first project but there are really better and more reliable ways to accomplish your goal. Lets say you just install the Tor bundle (including Vidalia and Privoxy). All you need now is a decent site ripper like HTTrack or Offline Explorer and set it to the proper proxy address (localhost / 127.0.0.1 Port 8118)
-
Your flawed approach aside this might help you a little: Firefox command line arguments No need for that F6 stuff at all. As said before you might be much more successful with _INetGetSource or _InetGet and a couple of string searches or regular expressions.
-
If WinExists("MAME32k") Then ... ElseIf ; <- This should throw a syntax error. It needs a statement. In case you mean Else then WinWaitClose("MAME32k") ; this would always immediately return 1 because Mame32k already doesn't exist Terminate() EndIf
-
Now that the latest beta supports console applications (many thanks) i'm wondering if there's a way to read keyboard input. Prompts like "Do you really want to ... (Y/N)" come to mind or even fully fledged console apps that work like nslookup or diskpart.
-
GuiCtrlCreateInput embed in a Run command?
ZoSTeR replied to schilbiz's topic in AutoIt General Help and Support
Currently your $s_Machine and $s_Process hold the control id. You have to query the value with GuiCtrlRead() in your OKButton func. $real_s_Machine = GuiCtrlRead($s_Machine) -
TC context Menu stops runing au3
ZoSTeR replied to Leagnus's topic in AutoIt General Help and Support
DllCall always seems to wait for a return value. Not directly related to your question but if you're trying to save the current TC tabs it might be better to use "Save Settings" (command 580) and then parse the wincmd.ini. And by the way, why not $tcPath = envGet("COMMANDER_PATH") -
Ok the actual problem is the 0x00 hex value that doesn't get passed on. Only thing i could think of is this: $buffer = StdoutRead($proc_Unrar,1) If $buffer = "" Then $buffer = Chr(0) Well this isn't elegant or effective at all. Any other suggestions?
-
I'm trying to read binary data from StdOut and write it to a file but it gets corrupted. Maybe im doing something wrong or StdOutRead isn't supposed to work with binary input. I tried various combinations with Binary() but without success. Here's a sample: #include <Constants.au3> Dim $proc_Unrar $fh_File01 = FileOpen("D:\OUT_STD.bin",18) $proc_Unrar = Run("Unrar.exe p -inul D:\BinaryData.rar", "", "", $STDERR_CHILD + $STDOUT_CHILD) While 1 $buffer = StdoutRead($proc_Unrar) $err_StdoutRead = @error If $err_StdoutRead Then ExitLoop FileWrite($fh_File01,$buffer) Wend FileClose($fh_File01) MsgBox(0, "Debug", "Exiting...")