FAQ
This is a list of frequently asked questions asked on the forums.
Other FAQs include:
- The one found in the AutoIt HelpFile. Much of it is about the transition from V2 to V3, but most is still relevant and should be a port of call, as well as this one.
How can I debug my script?
In SciTE
This one has a myriad of answers, but the most effective is to begin by using the SciTE4AutoIt3 Editor to create or edit scripts. This program is useful in debugging for the following reasons:
- Syntax highlighting allows for immediate viewing of any mistakes from unended script tags or quotes. This allows the scripter to visibly see the difference between the following portions of code.

And the correct version:

- Global syntax check built directly into the tools menu allows you to check an entire script for problems all at once.
- Built-in code tidying program that correctly indents untidy code and repairs messy scripts to allow them to be more readable. It also corrects problems with incorrectly capitalised function names and variables.
- Per-line trace insertion allows you to log every line of code executed to debug errors.
- Debug MsgBoxes or ConsoleWrites are able to be added anywhere in the script directly from SciTE. ConsoleWrite lines are less intrusive and prevent the added annoyance to the user of MsgBoxes.
You can also use AutoIt3Wrapper directive:
#AutoIt3Wrapper_Run_Debug_Mode=Y
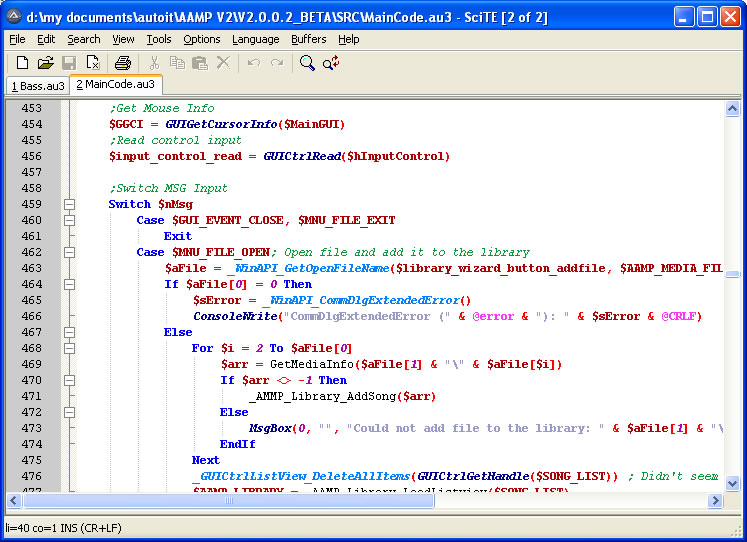
Using more code to check @error value
Nearly all functions will return something. Most of the time when a function fails, it returns 0 and sets @error. You can use this information not only to create scripts that handle for different errors but to debug and find out exactly why you are having problems. Check the help file for specific functions and their return values.
#include <MsgBoxConstants.au3>
_Example()
Func _Example()
Local $aFileList = _FileListToArray(@ScriptDir, 'NO_EXISTING_FILE.TEST')
If @error Then ; check if _FileListToArray() function return any error
MsgBox($MB_ICONERROR, '_FileListToArray', '@error = ' & @error & @CRLF & '@extended = ' & @extended) ; show error message
Return ; return / exit from function to prevent unexpected further error's
EndIf
For $iFile_idx = 1 To $aFileList[0]
FileRead($aFileList[$iFile_idx])
; any further code
Next
EndFunc ;==>_Example
Via the Tray icon
You can add this line at the top of your script (1 is on, 0 is off) to enable debugging from the tray icon.
AutoItSetOption ("TrayIconDebug", 1);0-off
Then if you run the script (uncompiled as an AU3 file), you can mouse over the AutoIt icon in the system tray (down by the clock) to display debugging information.
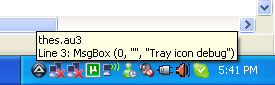
OutputDebugString native call
You can also debug a script on any computer by adding the following code to your script:
Func _MyDebug($sMessage, $iError = @error, $iExtended = @extended)
If $iError Or $iExtended Then
$sMessage &= '[ @error = ' & $iError & ' @extended = ' & $iExtended & ' ]'
EndIf
DllCall("kernel32.dll", "none", "OutputDebugString", "str", $sMessage)
Return SetError($iError, $iExtended, '')
EndFunc ;==>_MyDebug
Then, when you need to add a debug line, call it as necessary. Example:
_MyDebug("The value of Variable 1 at this time is " & $var1)
This debugging is completely transparent to the user, and is only viewable with a program such as DebugView from SysInternals. This method of debugging has the added advantage of being available to the developer in situations where is not acceptable or feasable to install SciTE on a client's unit.
Graphical debugger
Stumpii created a Graphical AutoIt Debugger, similar to Dev-C++'s debugging style.
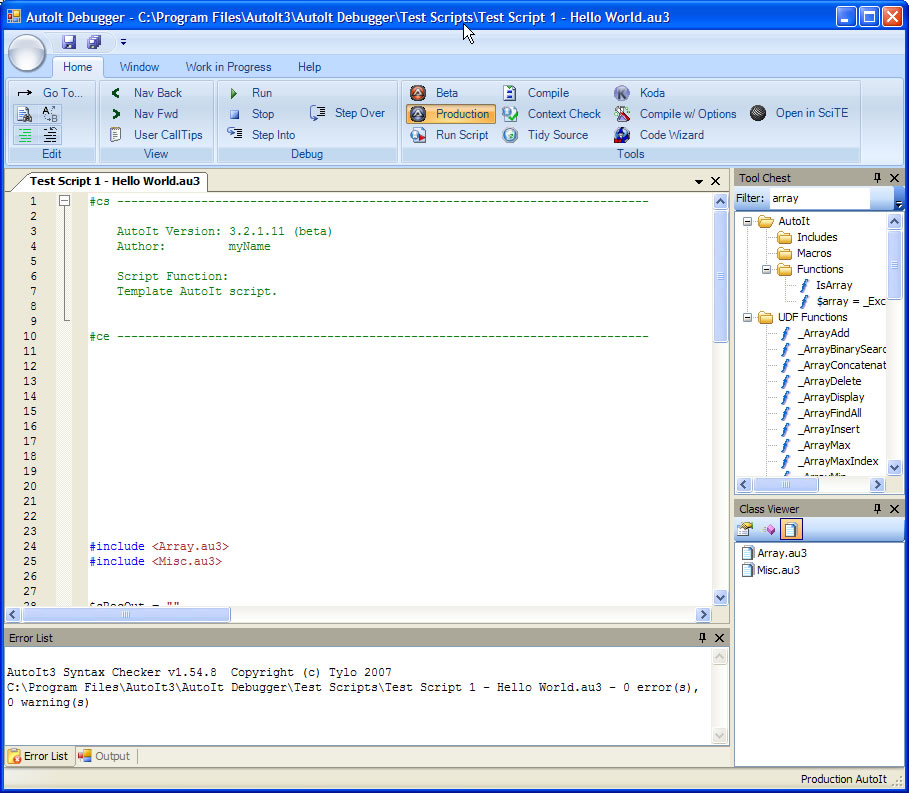
Another debugger for AutoIt
Heron created a Another debugger for AutoIt.
The latest version by asdf8 is here: https://www.autoitscript.com/forum/topic/103142-another-debugger-for-autoit/?do=findComment&comment=1303208
Other UDF to log/trace/script analyze
- ErrorLog (by mLipok) - Logs program activities and errors to different output locations.
- Log4a (by zorphnog) - Logging library loosely based upon the log4j and NLog libaries.
- Loga (by Danyfirex) - Simple logging library to keep track of code with an integrated console.
How can I run something that is not an exe file [.txt, .msi, .pdf,.jpg etc.] [or] How can I open a webpage in the default browser?
It was for this reason that the ShellExecute function was created. Here is one example:
ShellExecute("C:\autoitscripts\test.au3", "", "", "edit", @SW_MAXIMIZE)
You can also specify a web address this way:
ShellExecute("http://www.autoitscript.com/forum", "", "", "open")
If you normally are able to right-click the file and select print, then you can also print the file from AutoIt using this function:
ShellExecute("C:\boot.ini", "", "", "print")
If you wish to open the file with the default program, you can do as follows:
ShellExecute("C:\autoitscripts\test.au3")
If you wish your script to wait until the process is finished, you can use the ShellExecuteWait function with the same parameters.
Note: The default verb is the verb configured in the registry. If no verb is set as default in the registry then the "open" verb is used. If the "open" verb is not present then the first verb listed in the registry is used.
How can I prevent more than one copy of my script from running at once, or detect another copy of my script running?
_Singleton function
There are a few different ways to go about this. You can use a function called _Singleton to detect multiple instances of your script. An example of how to use this code:
#include <Misc.au3>
_Singleton("TheNameOfMyScript")
In this instance, the script will bring up some messages explaining to the user of the occurring events. This example will show you how the above function can be used in a real world application.
#include <Misc.au3>
#include <MsgBoxConstants.au3>
If _Singleton("MyScriptName", 1) = 0 Then
; If successful, running our script a second time should cause us to fall through here
MsgBox($MB_ICONERROR, "User Generated Error Message", "Error: This script is already running!")
Else
; We have detected that we are the only instance running, now we will run a second instance to display _Singleton's function!
MsgBox($MB_ICONINFORMATION, "Information!", "We are the first instance of this script, press OK to run another instance and trigger the error message!")
Switch @Compiled
Case 1
Run(FileGetShortName(@ScriptFullPath));when running an app, it's usually better to use its short name
Case 0
Run(FileGetShortName(@AutoItExe) & ' /AutoIt3ExecuteScript ' & FileGetShortName(@ScriptFullPath))
EndSwitch
Sleep(1000)
MsgBox($MB_ICONINFORMATION, "Information!", "We ran a second instance, you should have recieved an error message!", 5)
EndIf
Exit
_MutexExists function
Another method is to use _MutexExists.
#include <MsgBoxConstants.au3>
If _MutexExists("MydeswswScriptName") Then
; We know the script is already running. Let the user know.
MsgBox($MB_OK, "Script Name", "This script is already running. Using multiple copies of this script at the same time is unsupported!")
Exit
EndIf
;Function Author- Martin
Func _MutexExists($sOccurenceName)
Local $ERROR_ALREADY_EXISTS = 183, $handle, $lastError
$sOccurenceName = StringReplace($sOccurenceName, "", ""); to avoid error
$handle = DllCall("kernel32.dll", "int", "CreateMutex", "int", 0, "long", 1, "str", $sOccurenceName)
$lastError = DllCall("kernel32.dll", "int", "GetLastError")
Return $lastError[0] = $ERROR_ALREADY_EXISTS
EndFunc;==>_MutexExists
How can I run my script as a service?
This is also a question with multiple answers, and none of them are the only way to do it. The first question to ask yourself is whether or not you wish to install the service on other computers besides your own.
On your own computer - One time only
The easiest way to do this is to use Pirmasoft RunAsSvc. This program makes services easy to install and easy to remove when necessary.
On all computers that run your script
To do this you can use SRVANY.EXE and ServiceControl.au3. You can then use this code to install your script as a service:
#include "ServiceControl.au3"
$servicename = "MyServiceName"
_CreateService("", $servicename, "My AutoIt Script", "C:\Path_to_\srvany.exe", "LocalSystem", "", 0x110)
RegWrite("HKLM\SYSTEM\CurrentControlSet\Services\" & $servicename & "\Parameters", "Application", "REG_SZ", @ScriptFullPath)
Or use the following code to delete this service:
#include "ServiceControl.au3"
$servicename = "MyServiceName"
_DeleteService("", $servicename)
There is one caveat to setting up AutoIt as a service. If the service is not installed using the above code, it must have the "allow service to interact with the desktop" setting or else automation functions such as Control* or Win* functions will not function. To assure the service does indeed have this setting, use the following code:
RegWrite("HKLM\SYSTEM\CurrentControlSet\Services\[ServiceName]", "Type", "REG_DWORD", 0x110)
How can I create/start/stop or otherwise control a service?
There are two include libraries that are designed specifically to interact with services. These are the following:
- ServiceControl.au3 made by SumTingWong. Functionality:
- _StartService()
- _StopService()
- _ServiceExists()
- _ServiceRunning()
- _CreateService()
- _DeleteService()
- _NTServices.au3 made by CatchFish. Functionality:
- _ServiceStart()
- _ServiceStop()
- _ServiceStatus()
- _ServicePause()
How can I display a progress bar while copying files or directories?
There are a many different topics on how to do this. For more, just search the forums for Progress Copy + Progress.
- Copy with progress dialog... By ezztabi
- Yet another copy with progress... By SumTingWong
- _MultiFileCopy... By Oscar (German Forums)
- _FileCopy... By Jos
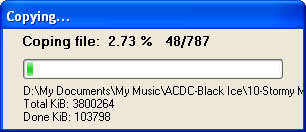
How can I make a hotkey that only works in my GUI?
It used to be quite tedious setting hotkeys to only work in your GUI. Now there is an easier way with the function GUISetAccelerators.
(From Helpfile example)
; A simple custom messagebox that uses the MessageLoop mode
#include <GUIConstantsEx.au3>
#include <MsgBoxConstants.au3>
GUICreate("Custom Msgbox", 210, 80)
GUICtrlCreateLabel("Please click a button!", 10, 10)
$YesID = GUICtrlCreateButton("Yes", 10, 50, 50, 20)
$NoID = GUICtrlCreateButton("No", 80, 50, 50, 20)
$ExitID = GUICtrlCreateButton("Exit", 150, 50, 50, 20)
; Set accelerators for Ctrl+y and Ctrl+n
Dim $AccelKeys[2][2]=[["^y", $YesID], ["^n", $NoID]]
GUISetAccelerators($AccelKeys)
GUISetState(); display the GUI
Do
$msg = GUIGetMsg()
Select
Case $msg = $YesID
MsgBox($MB_OK, "You clicked on", "Yes")
Case $msg = $NoID
MsgBox($MB_OK, "You clicked on", "No")
Case $msg = $ExitID
MsgBox($MB_OK, "You clicked on", "Exit")
Case $msg = $GUI_EVENT_CLOSE
MsgBox($MB_OK, "You clicked on", "Close")
EndSelect
Until $msg = $GUI_EVENT_CLOSE Or $msg = $ExitID
How can I perform an action while a key is held down?
You can use the _IsPressed() function to determine when a key is held down. The values that can be specified in this function are listed in the AutoIt Help File under User Defined Functions -> Misc Management -> _IsPressed. The following example will press the left mouse button while the k key is held down.
#include <Misc.au3>
$pressed = 0
While 1
If _IsPressed("4B") Then
If Not $pressed Then
ToolTip("K Key being held down")
MouseDown("left")
$pressed = 1
EndIf
Else
If $pressed Then
ToolTip("")
MouseUp("left")
$pressed = 0
EndIf
EndIf
Sleep(250)
WEnd
How can I run my script on a remote computer over the network?
The answer to this question depends on how much experience you have in networking. If the target system is a Windows system to which you have administrator access then you may use one of the following programs:
- PsExec from SysInternals
- BeyondExec from BeyondLogic
With either of these programs it is possible to launch any process on a remote system and even copy your script to the target computer before starting it. Neither these programs nor any others will work with Windows XP Home Edition unless you create a custom "command listener" that you manually install on the target system.
NOTE: Those of you with more advanced programming skills and a little imagination can figure out how to use the service control libraries and srvany.exe to do this same thing without either of the above mentioned programs.
How can I make a User Defined Function with optional parameters like the ones I see in the Help File?
You can specify optional parameters by giving them a default value in the Func declaration. An example of how this is done:
#include <MsgBoxConstants.au3>
Func testme($param1, $param2 = "nothing", $param3 = 5)
MsgBox($MB_OK, "", "Parameter one is required. The value of Parameter 1 is " & $param1 & @CRLF & _
"Parameter 2 is optional. The value of Parameter 2 is " & $param2 & @CRLF & _
"Parameter 3 is optional. The value of Parameter 3 is " & $param3)
EndFunc
If testme() is called with only one parameter [I.E. testme("test")] then the output is:
Parameter one is required. The value of Parameter 1 is test
Parameter 2 is optional. The value of Parameter 2 is nothing
Parameter 3 is optional. The value of Parameter 3 is 5
However, if the function is called with more than one parameter like this testme("test", "something"), then the output is
Parameter one is required. The value of Parameter 1 is test
Parameter 2 is optional. The value of Parameter 2 is something
Parameter 3 is optional. The value of Parameter 3 is 5
How can I make my script start every time windows starts?
You can use one of the following codes to allow your script to start with Windows:
RegWrite("HKLM\SOFTWARE\Microsoft\Windows\CurrentVersion\Run", "MyProgramName", "REG_SZ", @ScriptFullPath)
or
FileCreateShortcut(@ScriptFullPath, @StartupCommonDir & "\MyProgramName.lnk")
or place a Shortcut in the Startup folder, or the entire Executable. For Win8+ systems, use the Win+R keys to open a Run box, then "Shell:Startup" to access the Startup folder. Due to Windows User Account Control (UAC) restrictions, your script may require #RequireAdmin.
How can I have the script delete itself?
The following function will delete the running script or .exe once it has finished execution.
WARNING: Make a copy of your script before calling this function!!!
Func _SelfDelete($iDelay = 0)
Local $sCmdFile
FileDelete(@TempDir & "\scratch.bat")
$sCmdFile = 'ping -n ' & $iDelay & ' 127.0.0.1 > nul' & @CRLF _
& ':loop' & @CRLF _
& 'del "' & @ScriptFullPath & '"' & @CRLF _
& 'if exist "' & @ScriptFullPath & '" goto loop' & @CRLF _
& 'del %0'
FileWrite(@TempDir & "\scratch.bat", $sCmdFile)
Run(@TempDir & "\scratch.bat", @TempDir, @SW_HIDE)
EndFunc;==>_SelfDelete
How can I create a clickable website hyperlink in my gui?
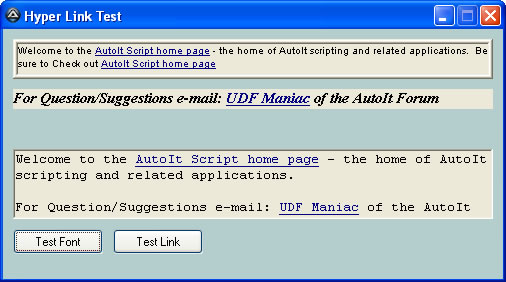
Gary Frost has made great advances in this area and has provided a UDF here (the UDF was removed from the post) to help with doing this.
How can I change the screen resolution / refresh rate / color depth?
ChangeResolution.au3 is a library function created to make changes to these settings.
How can I get the screen resolution in multiple monitor setups?
The following code was worked out by Larry to determine the total screen resolution of multiple monitors:
#include <MsgBoxConstants.au3>
$aTSR = _GetTotalScreenResolution()
MsgBox($MB_OK, "Total Screen Resolution", "Width = " & $aTSR[0] & @CRLF & _
"Height = " & $aTSR[1])
;Original code by Larry.
;Edited by BrettF
Func _GetTotalScreenResolution()
Local $aRet[2]
Global Const $SM_VIRTUALWIDTH = 78
Global Const $SM_VIRTUALHEIGHT = 79
$VirtualDesktopWidth = DllCall("user32.dll", "int", "GetSystemMetrics", "int", $SM_VIRTUALWIDTH)
$aRet[0] = $VirtualDesktopWidth[0]
$VirtualDesktopHeight = DllCall("user32.dll", "int", "GetSystemMetrics", "int", $SM_VIRTUALHEIGHT)
$aRet[1] = $VirtualDesktopHeight[0]
Return $aRet
EndFunc
How can I register a file type with my program [or] How can I make files with a certain extension open in my program?
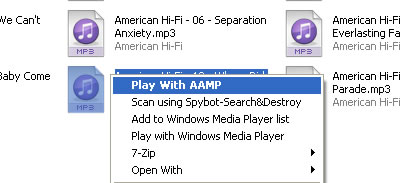
File registration can be a tricky business for those who have not done it before. The first thing to be done is to modify your script to allow it to accept files from the command line. Here is one example of how to do this:
#include <MsgBoxConstants.au3>
;$cmdline[0] is the number of parameters passed
If $cmdline[0] <> 0 Then
$filename = $cmdline[1]
;Do something with the file here
MsgBox($MB_OK, "UXYFixer", 'The file name passed to the command line is "' & $filename & '"')
Else
; We did not get any command line parameters.
; If this is a command line only program, you would want to
; alert the user that the command line parameters were incorrect.
; If this is a GUI program (like a notepad program), you would
; want to simply continue from here without opening a file.
MsgBox($MB_OK, "UXYFixer", 'Command line parameters incorrect.' & @CRLF & 'Command line usage: "' & @ScriptName & '" "file to process"')
EndIf
After your script is ready to accept files, you can begin to register the file type you need with your program. To prevent errors, this-is-me has created a UDF that will allow you to do this easily:
Here is an example of how to register and unregister a file extension using this UDF:
#include "FileRegister.au3"
;================================================
;
; Description: FileRegister($ext, $cmd, $verb [, $def [, $icon = "" [, $desc = "" ]]])
; Registers a file type in Explorer
;
; Parameter(s): $ext - File Extension without period eg. "zip"
; $cmd - Program path with arguments eg. '"C:\test\testprog.exe" "%1"'
; (%1 is 1st argument, %2 is 2nd, etc.)
; $verb - Name of action to perform on file
; eg. "Open with ProgramName" or "Extract Files"
; $def - Action is the default action for this filetype
; (1 for true 0 for false)
; If the file is not already associated, this will be the default.
; $icon - Default icon for filetype including resource # if needed
; eg. "C:\test\testprog.exe,0" or "C:\test\filetype.ico"
; $desc - File Description eg. "Zip File" or "ProgramName Document"
;
;================================================
FileRegister("uxy", '"' & @ScriptFullPath & '" "%1"', "Open in UXYFixer", 1, @ScriptFullPath & ',0', "UXYFixer Document")
;========================================
;
; Description: FileUnRegister($ext, $verb)
; UnRegisters a verb for a file type in Explorer
; Parameter(s): $ext - File Extension without period eg. "zip"
; $verb - Name of file action to remove
; eg. "Open with ProgramName" or "Extract Files"
;
;========================================
FileUnRegister("uxy", "Open in UXYFixer")
Download here: FileRegister.au3
Why doesn't my combobox (GUICtrlCreateCombo) show a dropdown list when clicked?
When using GUICtrlCreateCombo be sure to enter the desired height for your combobox list in the "height" parameter. Windows XP automatically selects an appropriate height for combo boxes, but other versions of Windows usually do not.
$combo = GUICtrlCreateCombo("",10,10,200,20)
Would correctly be changed to:
$combo = GUICtrlCreateCombo("",10,10,200,200)
Why isn't my thread getting any replies?
Asking the right question right
Did you give a good description of the problem? If your title or explanation of the issue is not descriptive, users are likely to bypass your issue instead of helping. Post titles such as "Help Me", "I Have A Problem", "Question", "Help me fix my code", "This code doesn't work" or similarly worded titles will not readily draw forum users to your post. Experienced users (which are your best hope of resolving the issue) will often skip your post altogether in cases like this. An example of a post title descriptive enough to attract users to assist you is "Problem with WinWaitClose" or "Loop never ends".
Example for reproduction
Did you post example code? If you have not posted an example of the code you are having an issue with, then you will not recieve support. When posting a non-working script, please do so in the smallest amount of stand-alone code possible. If the code you post cannot run by itself on another person's computer, they will not be able to recreate the issue.
Information about Environment
Did you provide any information about your AutoIt Development Environment ?
#include <APILocaleConstants.au3>
#include <WinAPILocale.au3>
; Version: 1.01. AutoIt: V3.3.14.2
; Retrieve the recommended information of the current system when posting a support question.
Local $sSystemInfo = 'I have a valid AutoIt support question and kindly provided the details of my system:' & _
@CRLF & @CRLF & 'AutoIt Version: V' & @AutoItVersion & ' [' & (@AutoItX64 ? 'X64' : 'X32') & ']' & _
@CRLF & 'Windows Version: ' & @OSVersion & '/' & (@OSServicePack <> "" ? @OSServicePack : _
RegRead("HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion", "ReleaseId")) & _
' [' & @OSArch & ']' & @CRLF & 'Language: ' & _GetOSLanguage() & ' (' & @OSLang & ')' & @CRLF & @CRLF
ClipPut($sSystemInfo)
MsgBox(4096, 'This info has been copied to the clipboard. Use Ctrl + V to retrieve it.', $sSystemInfo)
Func _GetOSLanguage()
Return _WinAPI_GetLocaleInfo(_WinAPI_GetUserDefaultLCID(), $LOCALE_SLANGUAGE)
EndFunc ;==>_GetOSLanguage
Speak English and speak it right
Did you use proper English? Here are guidelines for posting properly in the English language:
- Use proper case. THIS IS CONSIDERED YELLING. If you post in ALL UPPERCASE or all lowercase then you will not be taken seriously.
- Use proper punctuation. Complete sentences need only one trailing punctuation mark. Twelve exclamation points after every sentence will not get you an answer more quickly (just the opposite) Writing a sentence without simple punctuation such as commas reflects badly on your attention to detail, and is considered a good judge of the poster's coding style. If you cannot summon the intellect to write a sentence with correct punctuation, you will most likely miss simple coding mistakes such as unterminated quotes.
Breaking forum rules, law, modesty, or common sense
Show common sense by following the forum rules, http://www.autoitscript.com/forum/topic/169097-forum-rules/, as well as generally accepted forum etiquette. Repeated failure to do so will result in sanctions by the Moderation team as it ruins the experience for everyone.
Why does the Ctrl key get stuck down after I run my script?
Keys virtually stuck
It could equally be the Shift or the Alt key.
If you use Send in a script and you have a problem with keys being stuck down then Send is the most likely culprit. A similar problem can occur with BlockInput. The solution is generally quite simple. If there is a key like Shift or Alt held down at the start of the Send sequence, but that key is released by the time the Send sequence finishes then the key will get 'stuck' down. As an example of a solution, you could replace the Send function in your script with the _SendEx function below.
The _SendEx function waits for the Shift, Alt and Ctrl keys to be released or pops up a warning if the $warn parameter is not an empty string. Therefore it is not intended to be used when one of these modifier keys has been set to be down using any combination of {ALTDOWN}, {SHIFTDOWN} and {ALTDOWN}.
#include < Misc.au3 >
#include <MsgBoxConstants.au3>
;Send the string $ss after the Shift Alt and Ctrl keys are released. Optionally give a warning after 1 sec if any of those keys are still down.
;Requires misc.au3 to be included in the script for the _IsPressed function.
Func _SendEx($ss, $warn = "")
Local $iT = TimerInit()
While _IsPressed("10") Or _IsPressed("11") Or _IsPressed("12")
If $warn <> "" And TimerDiff($iT) > 1000 Then
MsgBox($MB_TOPMOST, "Warning", $warn)
EndIf
Sleep(50)
WEnd
Send($ss)
EndFunc;==>_SendEx
Key sequence
This sequence of keys is also working fine (including in AutoItX).
Send("{CTRLDOWN}")
Send("a")
Send("{CTRLUP}")
General unstuck method
Shilbiz also discovered that the following can 'clear' locked down keys.
ControlSend("", "", "", "text", 0)
How can I use Pixel functions?
Using a color change as an event
The following is an example of using the pixel to check if the colour has changed in a specific region.
#include <MsgBoxConstants.au3>
Local $PixelCheck = 0, $NewCheck
$PixelCheck = PixelCheckSum(40, 50, 60, 70); Get the checksum for this area.
While 1; Keep going
$NewCheck = PixelCheckSum(40, 50, 60, 70)
If $PixelCheck <> $NewCheck Then
; The old pixel checksum and the new one are different.
$PixelCheck = $NewCheck; Update the $PixelCheck to the new value
; Let us know it has changed. Change this to what you want to happen when the colour in the region changes.
Msgbox($MB_ICONWARNING,"PixelChecksum","Pixel region has changed !")
EndIf
Sleep(50); Just to give the CPU a bit of a break.
Wend
Searching for a specific pixel color
This is an example of searching for a specific pixel (red), both pure red (0xFF0000) and red in a few different shades.
#include <MsgBoxConstants.au3>
; Find a pure red pixel in the range 0,0-20,300
$coord = PixelSearch( 0, 0, 20, 300, 0xFF0000 )
If Not @error Then
MsgBox($MB_OK, "X and Y are:", $coord[0] & "," & $coord[1])
EndIf
; Find a pure red pixel or a red pixel within 10 shades variations of pure red
$coord = PixelSearch( 0, 0, 20, 300, 0xFF0000, 10 )
If Not @error Then
MsgBox($MB_OK, "X and Y are:", $coord[0] & "," & $coord[1])
EndIf
Why doesn't my script work on a locked workstation?
On a locked station any window will never be active (active is only dialog with text "Press Ctrl+Alt+Del"). In Windows locked state applications run hidden (behind that visible dialog) and do not have focus and active status. So generally don't use Send() MouseClick() WinActivate() WinWaitActive() WinActive() etc. Instead use ControlSend() ControlSetText() ControlClick() WinWait() WinExists() WinMenuSelectItem() etc. Doing so allows you to interact with an application regardless of whether it is active or not. It's possible to run such a script from scheduler on locked Windows stations.
Where can I learn AutoIt? Are there any tutorials?
AutoIt is a constantly growing and evolving language with a diverse and engaged community of volunteers. This wiki, along with the official forum, will always be your best place for answers to questions as well as examples and tutorials. With this in mind, below are a few resources that can also help you as you become familiar with the language:
AutoIt 1-2-3, an interactive classroom by Valuater: http://www.autoitscript.com/forum/index.php?showtopic=21048
BrettF's updated AutoIt tutorial: http://www.autoitscript.com/forum/index.php?showtopic=84960
"Learn To Program Using FREE Tools with AutoIt" PDF Book by Jfish Learn To Program Using FREE Tools with AutoIt
YouTube has a number of videos available: YouTube AutoIt tutorials list
As always, when asking questions about a video or script you saw on an external source, please ensure you are adhering to the forum rules.
Why does my script no longer decompile?
Decompilation is no longer supported, and is only available for the older versions of AutoIt (Version 3.2.5.1 and earlier compiled scripts).
Please do not post on this topic; see the Decompiling FAQ for more information. Topics discussing decompilation of scripts will be locked immediately. Posting the source of a decompiled script with lead to immediate sanctions from the Moderation team, as it directly violates AutoIt EULA (End-User-License-Agreement).
You may not reverse engineer or disassemble the SOFTWARE PRODUCT or compiled scripts that were created with the SOFTWARE PRODUCT.
How can I get a window handle when all I have is a PID?
Refer to the following example showing converting and use when manipulating the window. The function is based on work by Smoke_N/Hubertus and Helge.
;Run process
$iPID = Run("Notepad.exe")
;Allow window to initialize...
Sleep (500)
;Get HWND.
$hWnd = _GetHwndFromPID($iPID)
;Maximize
WinSetState($hWnd, "", @SW_MAXIMIZE)
;Wait 2 seconds
Sleep(2000)
;Minimize
WinSetState($hWnd, "", @SW_MINIMIZE)
;Wait 2 seconds
Sleep(2000)
;Restore window
WinSetState($hWnd, "", @SW_RESTORE)
;Wait 2 seconds
Sleep(2000)
;Move top left corner of screen, and resize to 800x600
WinMove($hWnd, "", 0, 0, 800, 600)
;Wait 2 seconds
Sleep(2000)
;Calculate Center of screen.
$x = (@DesktopWidth / 2) - 400; Desktop width divided by 2, then minus half the width of the window
$y = (@DesktopHeight / 2) - 300; Desktop height divided by 2, then minus half the height of the window
;Move to center of screen
WinMove($hWnd, "", $x, $y)
;Wait 2 seconds
Sleep(2000)
;Close notepad
WinClose($hWnd)
;Function for getting HWND from PID
Func _GetHwndFromPID($PID)
$hWnd = 0
$winlist = WinList()
Do
For $i = 1 To $winlist[0][0]
If $winlist[$i][0] <> "" Then
$iPID2 = WinGetProcess($winlist[$i][1])
If $iPID2 = $PID Then
$hWnd = $winlist[$i][1]
ExitLoop
EndIf
EndIf
Next
Until $hWnd <> 0
Return $hWnd
EndFunc;==>_GetHwndFromPID
How can I use single or double quotes in strings?
It is a fairly simple concept once you get the basics down. Basically there are a few different ways to go about mixing quotes in strings.
A string in AutoIt can be encased in either single(') or double (") quotes. So if you want to add only one type of quote into your string, your first port of call is to use the other type of quote to encase the string. Like so:
#include <MsgBoxConstants.au3>
$var = "I am a 'quote' inside the string"
MsgBox ($MB_OK, "Test 1", $var)
$var = 'I wish I could be a "quote" inside the string!'
MsgBox ($MB_OK, "Test 1", $var)
If you have to have both types of quotes in the string, the easiest way is to escape the quote ending the string as so to speak. To do this, use two quotes instead of one. Like so:
#include <MsgBoxConstants.au3>
$var = 'I am a single ''quote'' inside the string made using "single quote!"'
MsgBox ($MB_OK, "Test 1", $var)
When should I bump my threads?
As a courtesy to other users you should only bump your post once in a 24 hour period. Doing this allows all users the chance to get helped equally. Also refer to Why isn't my thread getting any replies?, as this will help you get replies.
How can I protect my code from decompilation?
The fact of the matter is you can't fully protect your code. AutoIt is an interpreted language, so all scripts are interpreted, and that script has to get stored somewhere. There is still hope though. You can take certain measures to make the decompiled code less usable to the person that decompiled it. The first step is to obfuscate your code. This causes the code to become less readable. Basically variables and functions are renamed, making it very hard to make head or tail of what is what.
Please see the Decompiling FAQ for more information.
How can I decompile my AutoIt EXEs?
You cannot decompile your AutoIt compiled scripts unless it was compiled with AutoIt 3.2.5.1 or earlier.
In order to be able to recover your script from the compiled executable, you can use the following:
;Original Author SmokeN
If $CMDLINE[0] Then
If $CMDLINE[1] == "/SOURCE" AND $CMDLINE[2] == "MYPASSWORD" Then
FileInstall("ThisSCript.au3", @ScriptDir & "\DecompiledScript.au3")
EndIf
EndIf
Then if you run the compiled script with the following command line parameters your source will be extracted.
myscript.exe /SOURCE MYPASSWORD
What is proper forum etiquette? What rules are there for the forum?
Proper forum etiquette will ensure you will stay a member of these forums. So try to follow these rules. Also see this FAQ post, as you should follow what is outlined there as well.
- Use proper English. MSN speak and the like makes it very hard to understand what you want. Keep posts in proper case because UPPERCASE is considered yelling and lowercase makes you look stupid. Don't use more punctuation marks than necessary. For example ?!!!? and ????? is completely unnecessary.
- Before posting help with a script, make sure you follow these steps:
- Search for your issue.
- There are many thousands of posts on this forum, and chances are your question has been asked before. Make sure to use a variety of terms to maximize your results.
- Read the helpfile
- Most if not all things in the help file will have an example (native functions) or two. Give them a go to try see how the functions work. Most of the time it helps!
- Give it a go
- Seriously, if you can't be bothered to give it a go, then why should we be bothered to help you? If you feel you can't do it and you don't try then how will you ever know? We always prefer that you give it a go because you can learn from your mistakes and hopefully understand it better.
- Post your problem
- Make sure you post in the correct forum.
- AutoIt Specific
- General Help and Support (Most support questions)
- GUI Support (Support for the Graphical User Interface)
- AutoItX Support (Support for the COM, DLL, PowerShell and .NET add-ons for AutoIt)
- Non-AutoIt
- Chat (General chat for Active Members. Not accessible to guests or search engines)
- Developer General Discussion (General development and scripting discussions)
- Test Posting Messages (This forum is emptied of all topics and posts from time to time)
- Language Specific Discussion (Common IT Admin-related languages have their own forums. Feel free to post about any other language in the Misc forum)
- IT Administration (Discussions about general IT related topics, administration, management and deployment)
- AutoIt Specific
- Include a detailed description in the content of the post, and a detailed title
- Titles such as 'Help' and 'What's wrong?' are not acceptable. To get the most help with your problem, be as descriptive as you can in the title, but keep in mind your title cannot be too long.
- Make sure you also include a descriptive question. Just saying 'what is wrong with the following' is also not acceptable, as we will have no idea what the problem is. Describe what you think is wrong and what is not working. It makes our job easier.
- Always include your code
- It shows us that you have tried and gives us a head start with something to play with.
- Make sure you post in the correct forum.
- Search for your issue.
- Usually asking for a script is not taken too well, within reason. Keep in mind that this is a support forum, so please acknowledge that. We are here to help you with your scripts, not to spoon-feed code to you. If you do need something written for you, maybe try RentACoder, as they are more suited to requests.
- Don't PM other members asking for them to look at threads of to help you unless they request it. It is actually quite rude and annoying to receive PMs of that nature.
- Lurk a little before you dive right in. Read a number of posts, or check out the group's archives. Get a feel for the tone of the forum so you can participate accordingly.
- Say online exactly what you would say in person. In other words, if you wouldn't say it to the person's face in front of your Grandmother, you shouldn't type it here.
- Remember your face doesn't show. Words alone can convey sentiment, but without benefit of inflection or facial expression, they can be misconstrued. Use descriptive wording, emoticons or .gifs to ensure your meaning is clear. By the same token, don't jump to conclusions about another person's intent in posting an unclear comment. When in doubt, ask for clarification.
- Be respectful. Internet etiquette is similar to standard etiquette in this area. Appreciate that your opinion is one of many. You can disagree with another person without being disrespectful or rude to other people.
- Ignore Trolls. If you engage in conversation with one, you'll raise your blood pressure and empower the troll. You can't win a flame war, and you can't sway a troll's opinion. Often, they don't even care about the subject. They live for the conflict and nothing more. Trolls are common and not worthy of your time. Ignore their posts no matter how inflammatory and eventually they'll get bored and move on.
- When you have found an answer for your question:
- Do not edit your post to be blank or to have worthless information. People may be searching for the same issue you have, so be courteous to them by leaving your question there.
- It is also optional to add the word [SOLVED] into the title, as well as selecting the post that best answered your question. It can make the lives of people searching easier.
- Finally follow the rules:
Some general points taken from here.
Are there forums available in my local language?
Yes there are some available. Some forum members here participate in multiple forums.
Please note that these are independent, community-run message boards and not just a translation of this board.
How can I control (click, edit etc) an external (html) application?
You can use the control* functions of autoit see helpfile of use specific udf libraries
- IUIAutomation for multiple applications
- uia explained in detail
- chrome chrome.au3
- Internet explorer ie.au3
- firefox ff.au3 (End of life)
- java
- Selenium
- WebDriver UDF (IE, Chrome, FireFox)
You should use spy applications to identify objects like
- au3inf part of AutoIT
- simplespy in the zip
- uia spy
- jabsimplespy in examples section of the AutoIt forum
- control viewer
- inspect as part of windows SDK
- VisualUIAVerifyNative.exe as part of windows SDK (8.1)
If above spy tools are not identifying your object you could try commercial tools (trials) like
- ranorex spy
- Testcomplete
- HP UFT
And your last recognition can be bitmaps
- search for GDI
- search for findBMP
- search for imagesearch
Does AutoIt support database connections, such as SQL?
Yes! UDFs exist for several popular SQL solutions, including SQLite, Firebird, MySQL, MSSQL and ODBC connections for PostGreSQL and others.
For an illustrative list, see this link:
A DBF UDF also exists
How can I include some files into my compiled Autoit's EXE?
Use FileInstall() or ResourcesEx UDF from the forum Examples. Additional resources can also be included at compile time, click the "Res Add Files" tab.
How can I test if checkbox / radiobutton is checked?
Use this small function: If IsChecked($checkbox1) Then ...
; by Zedna
Func IsChecked($control)
Return BitAND(GUICtrlRead($control), $GUI_CHECKED) = $GUI_CHECKED
EndFunc ;==>IsChecked
How to use the {enter} key as a Tab?
Use Accelerator keys
; by Melba23
#include <GUIConstantsEx.au3>
#include <MsgBoxConstants.au3>
#include <WinAPI.au3>
$hGUI = GUICreate("Test", 500, 500)
$cInput_1 = GUICtrlCreateInput("", 10, 10, 200, 20)
$cInput_2 = GUICtrlCreateInput("", 10, 50, 200, 20)
$cInput_3 = GUICtrlCreateInput("", 10, 90, 200, 20)
$cButton_1 = GUICtrlCreateButton("Button 1", 250, 10, 80, 30)
$cButton_2 = GUICtrlCreateButton("Button 2", 250, 50, 80, 30)
$cButton_3 = GUICtrlCreateButton("Button 3", 250, 90, 80, 30)
$cEnter = GUICtrlCreateDummy()
GUISetState()
Local $aAccelKeys[2][2] = [["{ENTER}", $cEnter], ["{TAB}", $cEnter]] ; Make {TAB} an accelerator
GUISetAccelerators($aAccelKeys)
While 1
Switch GUIGetMsg()
Case $GUI_EVENT_CLOSE
Exit
Case $cEnter
Switch _WinAPI_GetFocus()
Case GUICtrlGetHandle($cInput_1)
; Check the input content
If checkEntry() = True Then
; Correct so move to next input
GUICtrlSetState($cInput_2, $GUI_FOCUS)
EndIf
; Not correct so stay in current input
Case GUICtrlGetHandle($cInput_2)
If checkEntry() = True Then
GUICtrlSetState($cInput_3, $GUI_FOCUS)
EndIf
Case GUICtrlGetHandle($cInput_3)
If checkEntry() = True Then
ConsoleWrite("End" & @CRLF)
EndIf
Case Else
GUISetAccelerators(0) ; Remove accelerator link
ControlSend($hGUI, "", "", "{TAB}") ; Send {TAB} to the GUI
GUISetAccelerators($aAccelKeys) ; Reset the accelerator
EndSwitch
EndSwitch
WEnd
Func checkEntry()
; Random chance of correct answer or fail
If Mod(@SEC, 2) = 0 Then
MsgBox($MB_SYSTEMMODAL, "Test Fail", "Press Enter to close this message box")
Return False
EndIf
Return True
EndFunc ;==>checkEntry
Why are my number sort results wrong?
AutoIt by default uses an alpha sort algorithm. This means that ABC is sorted correctly, but it sorts numbers as 1,10,2,22,3,31 where the user expects 1,2,3,10,22,31. This can be remedied by using a custom sort function. An example of such a custom number sort by Valuater appears below. Search the Forum for Number Sort and Natural Order String Comparison
; by Valuater
#include <array.au3>
$Array = StringSplit("2,5,3,4,6,1,8,9,7", ",")
_ArraySortNum($Array)
_ArrayDisplay($Array, "Sorted Array")
Func _ArraySortNum(ByRef $n_array, $i_descending = 0, $i_start = 1)
Local $i_ub = UBound($n_array)
For $i_count = $i_start To $i_ub - 2
Local $i_se = $i_count
If $i_descending Then
For $x_count = $i_count To $i_ub - 1
If Number($n_array[$i_se]) < Number($n_array[$x_count]) Then $i_se = $x_count
Next
Else
For $x_count = $i_count To $i_ub - 1
If Number($n_array[$i_se]) > Number($n_array[$x_count]) Then $i_se = $x_count
Next
EndIf
Local $i_hld = $n_array[$i_count]
$n_array[$i_count] = $n_array[$i_se]
$n_array[$i_se] = $i_hld
Next
EndFunc ;==>_ArraySortNum
How do I include AutoIt functions in a C# / C++ / VB programme? AutoItX
AutoIt is also supplied as a combined COM and DLL version of AutoIt called AutoItX that allows you to add the unique features of AutoIt to your own favourite scripting or programming languages! Info on AutoItX
From AutoItX.chm: AutoItX is a DLL version of AutoIt v3 that provides a subset of the features of AutoIt via an ActiveX/COM and DLL interface. This means that you can add AutoIt-like features to your favourite scripting and programming languages, e.g. VB, VBScript, Delphi, C, C++, Kixtart, and most other languages that support the use of DLLs.
Here are some C++ source code samples from various projects by Jon. Most are free to use but check any license files that accompany the downloads for details. All code was created in Microsoft Visual C (6 and 7) but most would probably compile under mingw32 as well. Download C++ examples for AutoItX
You don't need to regsvr it if you are using the c# library. The c# library uses the non-COM part of the DLL so it just needs to be in the same folder as the exe and add the assembly.dll to your project. From the documenation:
Using the Assembly from VB/C# within in Visual Studio is very easy:
- Add a reference to AutoItX3.Assembly.dll to your project
- Add a using AutoIt; statement in the files you want to use AutoIt functions
- Distribute your final executable with the files AutoItX3.Assembly.dll, AutoItX3.dll, AutoItX3_x64.dll.
- Write code like this C# example:
using AutoIt; ...
; by Jon
// Wow, this is C#!
AutoItX.Run("notepad.exe");
AutoItX.WinWaitActive("Untitled");
AutoItX.Send("I'm in notepad");
IntPtr winHandle = AutoItX.WinGetHandle("Untitled");
AutoItX.WinKill(winHandle);
From the AutoItX Help File (included in the default AutoIt install): AutoItX can be used as a standard DLL from any language capable of calling functions in external DLLs.
The following files are provided to allow you to use the DLL in C++:
- AutoItX3_DLL.h - C language header file showing the exported functions and parameters
- AutoItX3_DLL.lib - Microsoft format import library (x86)
- AutoItX3_x64_DLL.lib - Microsoft format import library (x64)
- AutoItX3.dll - The main AutoItX DLL (x86)
- AutoItX3_x64.dll - The main AutoItX DLL (x64)
How can I search an image in another image?
You can use the Pixel* functions of AutoIt. See the help file for more information and examples. You can also use one of the UDFs below; most of which use GDI:
Other libraries can be found that make use of a specific external dll but those above are considered "native" as no external dlls are required.
Multithreading
Multithreading basically is running multiple scripts/programs that have acces to the same variables, normally by sharing memory.
The AutoIt language is not threadsafe and multithreading is firmly on the NOT TO DO list
Multitasking and Multiprocessing are closely related, however, and multiple solutions have been explored:
- Multithread technical discussion
- Multithread with external DLL
- Multithread with external DLL 2
- Multi process
- Using C# - Host .NET
Test automation
Many people are looking for freeware tools to do test automation and cannot afford the commercial tools like HP LeanFT, Ranorex, Squish etc.
So how can AutoIt help you.
Out of the box install the base full zip package and start Scite.Exe as a simple IDE.
If you want to do more these links will help you.
Read FAQ 31 for the basic stuff on which technologies are readable and which spying tools there are
Read FAQ 38 for the bitmap stuff
Android can be reached thru ADB and Webdriver
iOS is limited to normal webdriver stuff thru WinHTTP library. So most likely you have to install additional iOS webdriver solutions
And after reading FAQ just ask your questions in the support forum
DPI Awareness
The following few links are related to DPI Awareness :
After much research and testing, DPI Awareness is solved in the following thread with all relevant information moved to the 1st post
- Writing DPI Awareness App - workaround
- Wrong Screen Resolution Displayed by Autoit Macros
- DPI Awareness - question
AutoIt+SciTE Portable Developer Environment
Recent discussion can be found here:
Does AutoIt have an agreed set of good practices ?
Wiki related resources: